Pandas DataFrame to Excel
You can save or write a DataFrame to an Excel File or a specific Sheet in the Excel file using pandas.DataFrame.to_excel()
method of DataFrame class.
In this tutorial, we shall learn how to write a Pandas DataFrame to an Excel File, with the help of well detailed example Python programs.
Prerequisite
The prerequisite to work with Excel file functions in pandas is that, you have to install openpyxl module. To install openpyxl using pip, run the following pip command.
pip install openpyxl
Examples
1. Write DataFrame to Excel File
You can write the DataFrame to Excel File without mentioning any sheet name. The step by step process is given below:
- Have your DataFrame ready. In this example we shall initialize a DataFrame with some rows and columns.
- Create an Excel Writer with the name of the output excel file, to which you would like to write our DataFrame.
- Call to_excel() function on the DataFrame with the Excel Writer passed as argument.
- Save the Excel file using save() method of Excel Writer.
Python Program
import pandas as pd
# create dataframe
df_marks = pd.DataFrame({'name': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]})
# create excel writer object
writer = pd.ExcelWriter('output.xlsx')
# write dataframe to excel
df_marks.to_excel(writer)
# save the excel
writer.save()
print('DataFrame is written successfully to Excel File.')
Output
Run the above program, and an excel file shall be created with the name specified while creating excel writer.
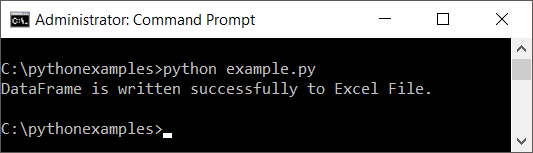
Output Excel File
Open the excel file, and you shall see the index, column labels and row data written to file.
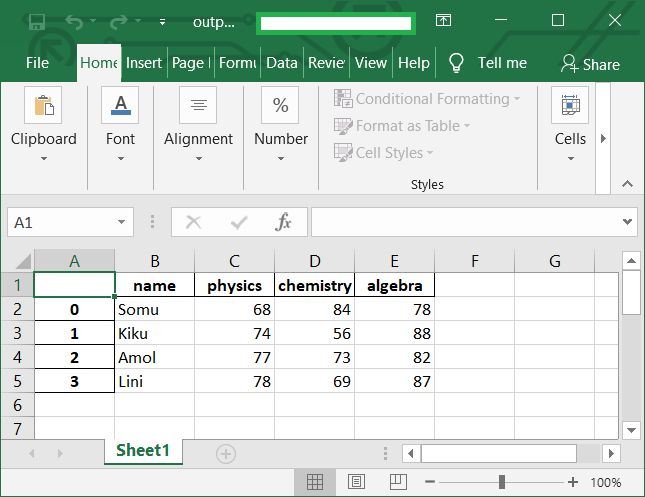
2. Write DataFrame to a specific Excel sheet
You can write the DataFrame to a specific Excel Sheet. The step by step process is:
- Have your DataFrame ready.
- Create an Excel Writer with the name of the desired output excel file.
- Call to_excel() function on the DataFrame with the writer and the name of the Excel Sheet passed as arguments.
- Save the Excel file using save() method of Excel Writer.
Python Program
import pandas as pd
# create dataframe
df_marks = pd.DataFrame({'name': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]})
# create excel writer
writer = pd.ExcelWriter('output.xlsx')
# write dataframe to excel sheet named 'marks'
df_marks.to_excel(writer, 'marks')
# save the excel file
writer.save()
print('DataFrame is written successfully to Excel Sheet.')
Run Program
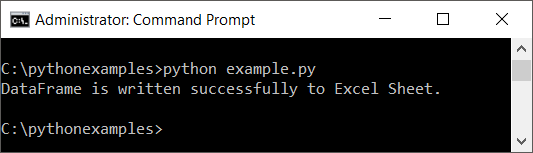
Output Excel File
Open the excel file. Please note the name of the excel sheet. It is named to the string we specified as second argument to to_excel() function.
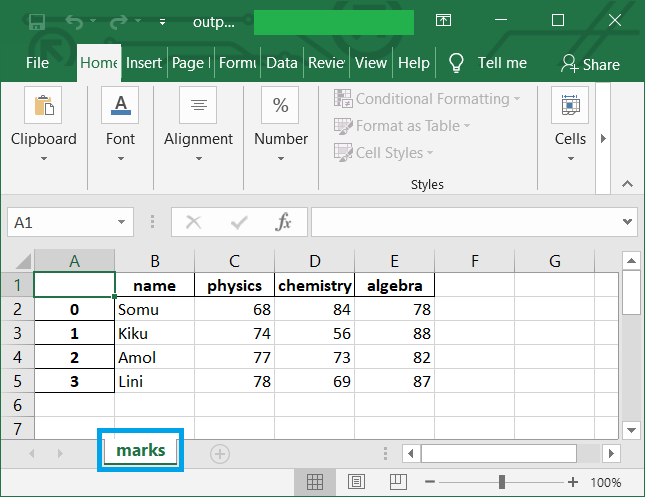
Summary
In this Pandas Tutorial, we learned how to write a Pandas DataFrame to Excel sheet, with the help of well detailed Python example programs.