Replace NaN values with Zero in Pandas DataFrame
You can replace NaN values with 0 in Pandas DataFrame using DataFrame.fillna() method. Pass zero as argument to fillna() method and call this method on the DataFrame in which you would like to replace NaN values with zero.
fillna() method returns new DataFrame with NaN values replaced by specified value.
Sample Code Snippet
Following is a sample code snippet to replace NaN values with 0.
df = df.fillna(0)
Examples
1. Replace NaN values with 0 in DataFrame
In the following Python program, we take a DataFrame with some of the values as NaN (numpy.nan). Then we will use fillna() method to replace these numpy.nan values with zero.
Python Program
import pandas as pd
import numpy as np
df = pd.DataFrame(
[[np.nan, 72, 67],
[23, 78, 62],
[32, 74, np.nan],
[np.nan, 54, 76]],
columns=['a', 'b', 'c'])
print('Original DataFrame\n', df)
df = df.fillna(0)
print('\nModified DataFrame\n', df)
Output
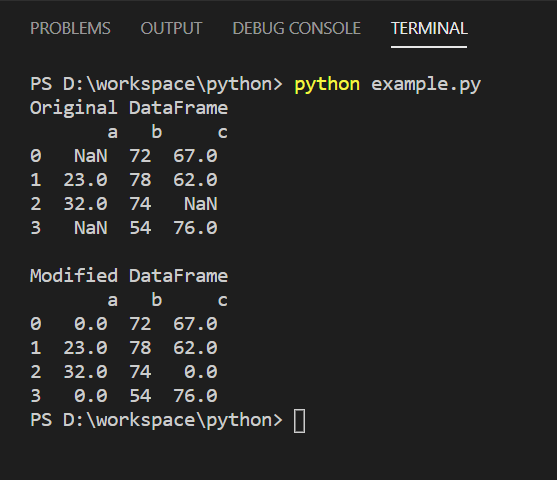
All the NaN values across the DataFrame are replaced with 0.
2. Replace NaN values with 0 in specified columns in DataFrame
You can also replace NaN values with 0, only in specific columns. Following example program demonstrates how to replace numpy.nan values with 0 for column ‘a‘.
Python Program
import pandas as pd
import numpy as np
df = pd.DataFrame(
[[np.nan, 72, 67],
[23, 78, 62],
[32, 74, np.nan],
[np.nan, 54, 76]],
columns=['a', 'b', 'c'])
print('Original DataFrame\n', df)
df['a'] = df['a'].fillna(0)
print('\nModified DataFrame\n', df)
Output
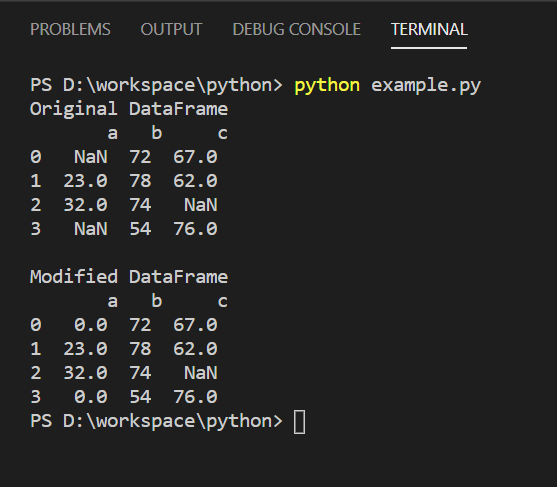
Summary
In this tutorial of Python Examples, we learned how to replace NaN values with 0 in DataFrame.