Pandas Series.to_csv() Tutorial
Pandas Series.to_csv() Tutorial
In this tutorial, we'll explore the Series.to_csv()
method in Pandas, which is used to write the contents of a Pandas Series to a CSV (Comma-Separated Values) file, with well detailed example programs.
The syntax of Series.to_csv()
is
Series.to_csv(path_or_buf=None, sep=', ', na_rep='', float_format=None, columns=None, header=True, index=True, index_label=None, mode='w', encoding=None, compression='infer', quoting=None, quotechar='"', lineterminator=None, chunksize=None, date_format=None, doublequote=True, escapechar=None, decimal='.', errors='strict', storage_options=None)
where
Parameter | Description |
---|---|
path_or_buf | File path or object, if None , writes to system sys.stdout . |
sep | String of length 1, default is ',' for comma-separated values. |
na_rep | String representing NaN , default is an empty string. |
float_format | String formatting for floating-point numbers. |
columns | Columns to write, default is None to write all columns. |
header | Write out the column names, default is True . |
index | Write row names (index), default is True . |
index_label | Column label for index column(s), default is None . |
mode | File mode, default is 'w' (write). |
encoding | Encoding to use for UTF when writing, default is None . |
compression | Compression mode, default is 'infer' . |
quoting | Quoting style, default is None . |
quotecharstr | String of length 1. Character used to quote fields. |
line_terminator | Character to break lines on, default is None . |
chunksize | Rows to write at a time, default is None . |
date_format | Format to use when serializing dates, default is None . |
doublequote | Control quoting of quotechar inside a field, default is True . |
escapechar | Character to escape sep and quotechar when appropriate, default is None . |
decimal | Character recognized as decimal separator, default is '.' . |
errors | Specifies how encoding and decoding errors are to be handled. See the errors argument for open() for a full list of options. |
storage_options | Extra options that make sense for a particular storage connection. |
The Series.to_csv()
method is useful for exporting data from a Pandas Series to a CSV file for further analysis or sharing with others.
Examples for Series.to_csv()
1. Write Series to a CSV file
In this example, we'll use Series.to_csv()
to write a Pandas Series to a CSV file. The CSV file will include the index and column names.
Python Program
import pandas as pd
# Create a Series with decimal values
series = pd.Series({'A': 10, 'B': 20, 'C': 30, 'D': 40})
# Write the Series to a CSV file
series.to_csv('output.csv')
# Print a message
print("Series written to 'output.csv'")
Output
Series written to 'output.csv'
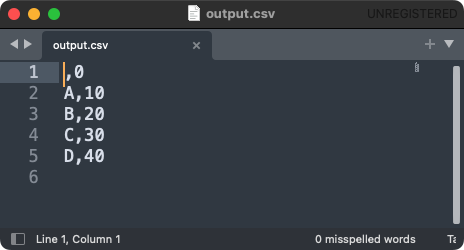
2. Write Series to a CSV file without header
In this example, we'll use Series.to_csv()
to write a Pandas Series to a CSV file without including the index and header.
Python Program
import pandas as pd
# Create a Series with decimal values
series = pd.Series({'A': 10, 'B': 20, 'C': 30, 'D': 40})
# Write the Series to a CSV file without header
series.to_csv('output_no_header.csv', header=False)
# Print a message
print("Series written to 'output_no_header.csv'")
Output
Series written to 'output_no_header.csv'
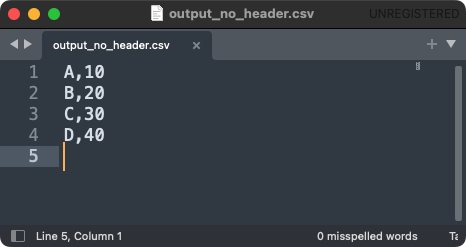
3. Write Series to a CSV file without index and header
In this example, we'll use Series.to_csv()
to write a Pandas Series to a CSV file without including the index and header.
Python Program
import pandas as pd
# Create a Series
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40]}
series = pd.Series(data)
# Write the Series to a CSV file without index and header
series.to_csv('output_no_index_header.csv', index=False, header=False)
# Print a message
print("Series written to 'output_no_index_header.csv'")
Output
Series written to 'output_no_index_header.csv'
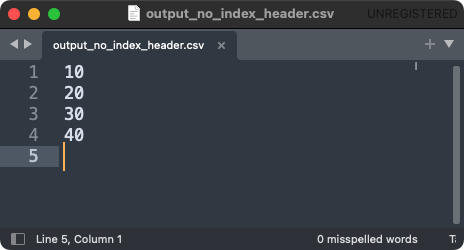
Summary
In this tutorial, we've covered the Series.to_csv()
method in Pandas, which is useful for exporting the contents of a Pandas Series to a CSV file. The method provides various options for customizing the CSV output, such as specifying the file path, separator, inclusion of index and header, and more.