MySQL Division Operator
MySQL Division Operator
The MySQL /
operator is used to divide one number or expression by another. This operator is essential for performing arithmetic calculations in SQL queries.
Syntax
SELECT column1 / column2 AS result
FROM table_name;
The /
operator has the following components:
column1
: The numerator column or value.column2
: The denominator column or value.result
: An alias for the resulting value.table_name
: The name of the table from which to retrieve the data.
Example MySQL Division Operator
Let's look at some examples of the MySQL /
operator:
Step 1: Using the Database
USE mydatabase;
This query sets the context to the database named mydatabase
.
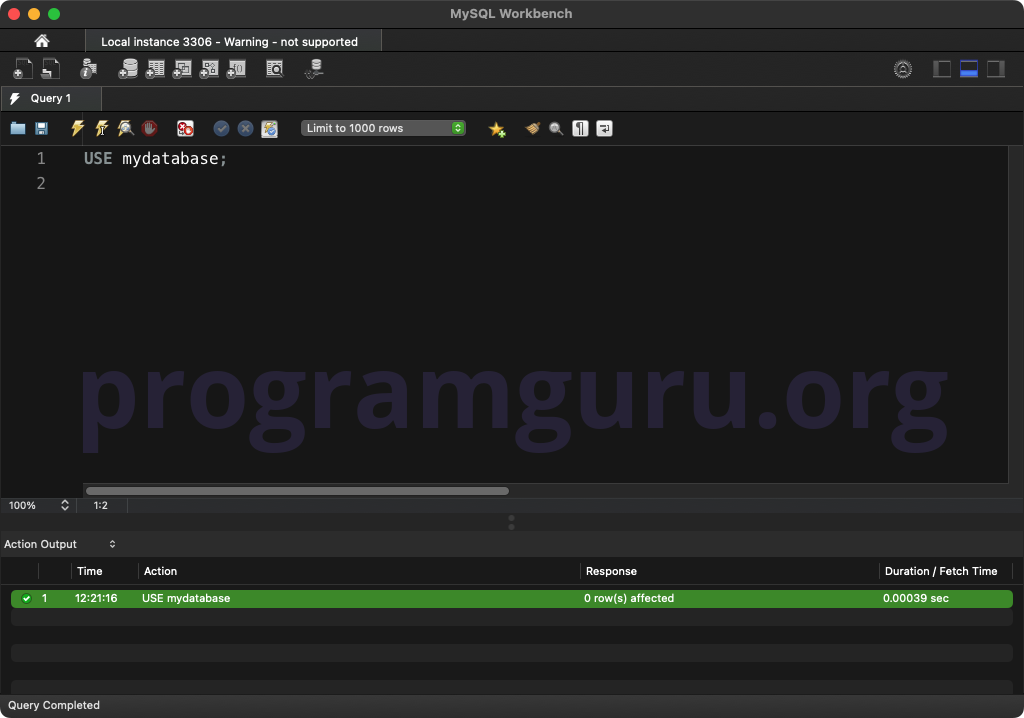
Step 2: Creating a Table
Create a table to work with:
CREATE TABLE sales (
id INT AUTO_INCREMENT PRIMARY KEY,
total_sales DECIMAL(10, 2) NOT NULL,
number_of_items INT NOT NULL
);
This query creates a table named sales
with columns for id
, total_sales
, and number_of_items
.
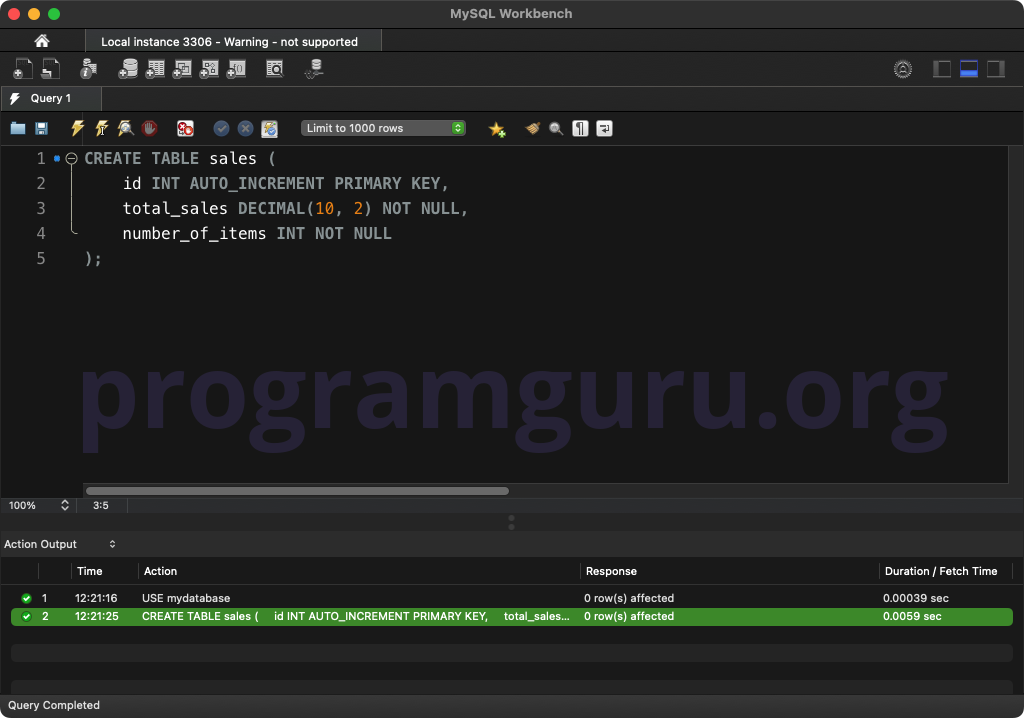
Step 3: Inserting Initial Rows
Insert some initial rows into the table:
INSERT INTO sales (total_sales, number_of_items)
VALUES (100.00, 10),
(150.00, 15),
(200.00, 20);
This query inserts three rows into the sales
table.
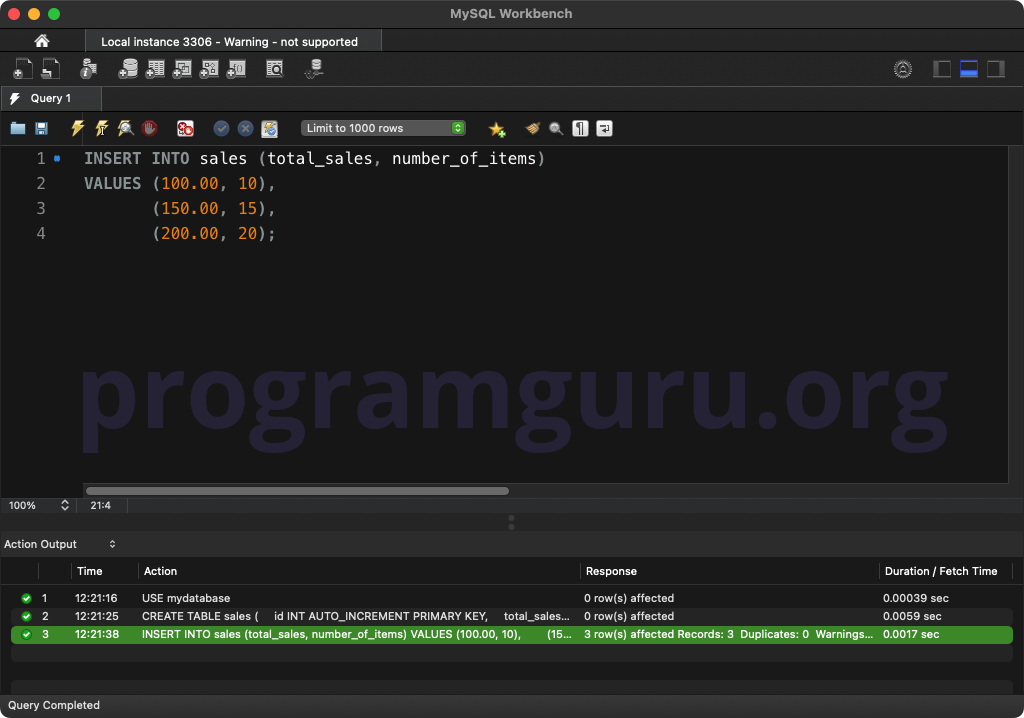
Step 4: Dividing Two Columns
Divide one column by another and display the result:
SELECT total_sales / number_of_items AS average_price_per_item
FROM sales;
This query divides the total_sales
column by the number_of_items
column and displays the result as average_price_per_item
.
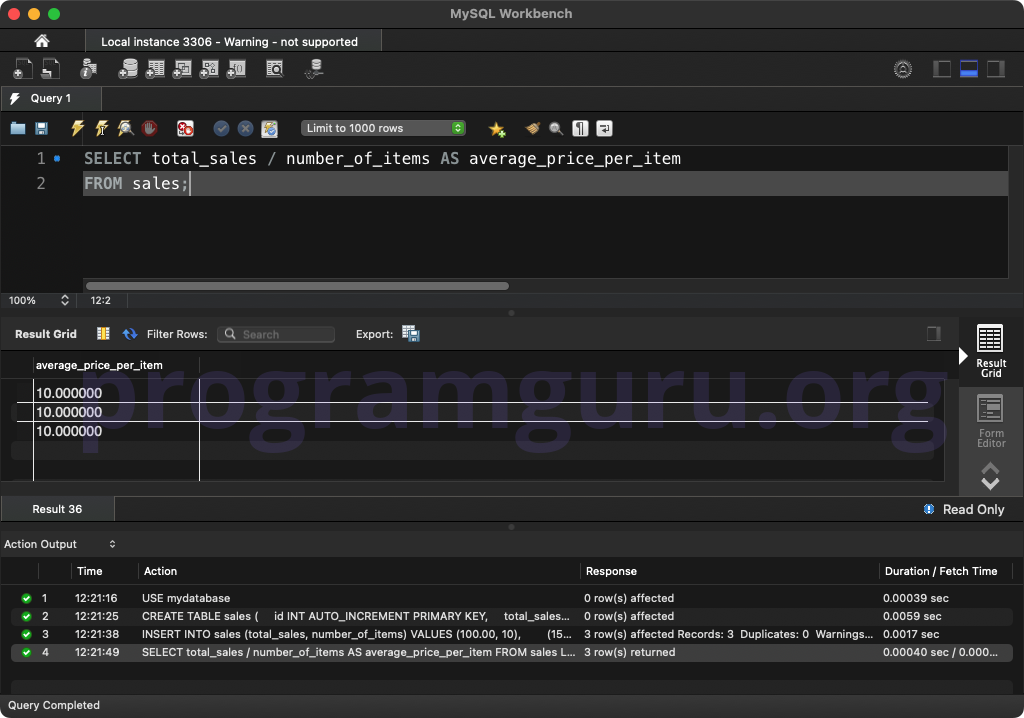
Step 5: Dividing a Column by a Constant
Divide a column by a constant value:
SELECT total_sales / 2 AS half_sales
FROM sales;
This query divides the total_sales
column by a constant value of 2, displaying the result as half_sales
.
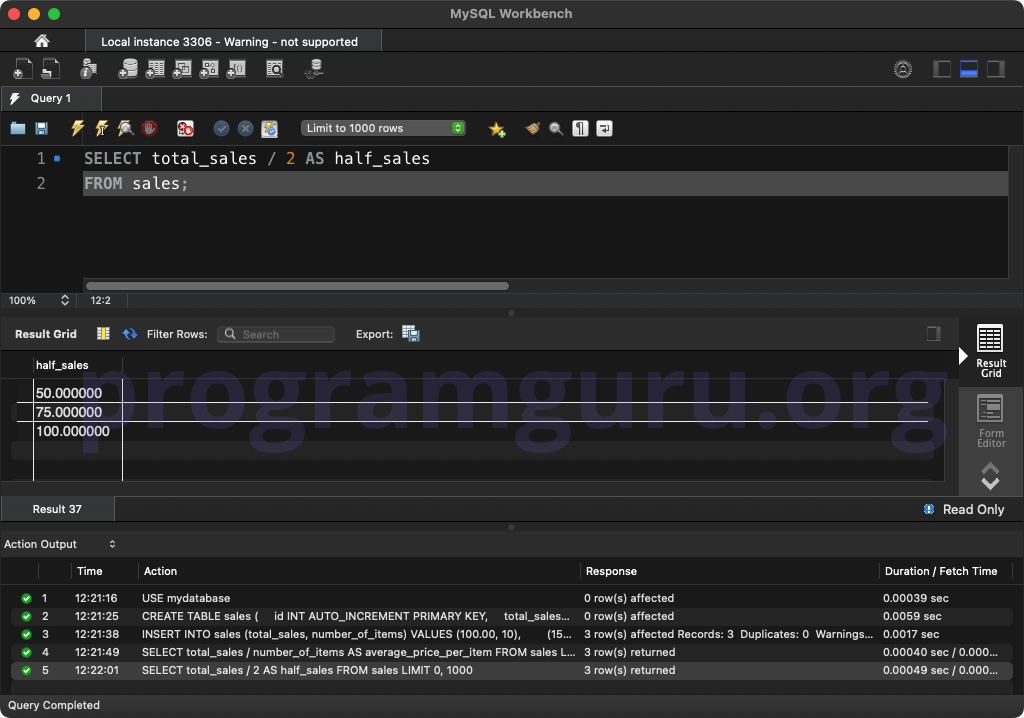
Step 6: Dividing Multiple Columns
Divide multiple columns and display the result:
SELECT total_sales / number_of_items / 2 AS adjusted_average_price
FROM sales;
This query divides the total_sales
column by the number_of_items
column and then by a constant value of 2, displaying the result as adjusted_average_price
.
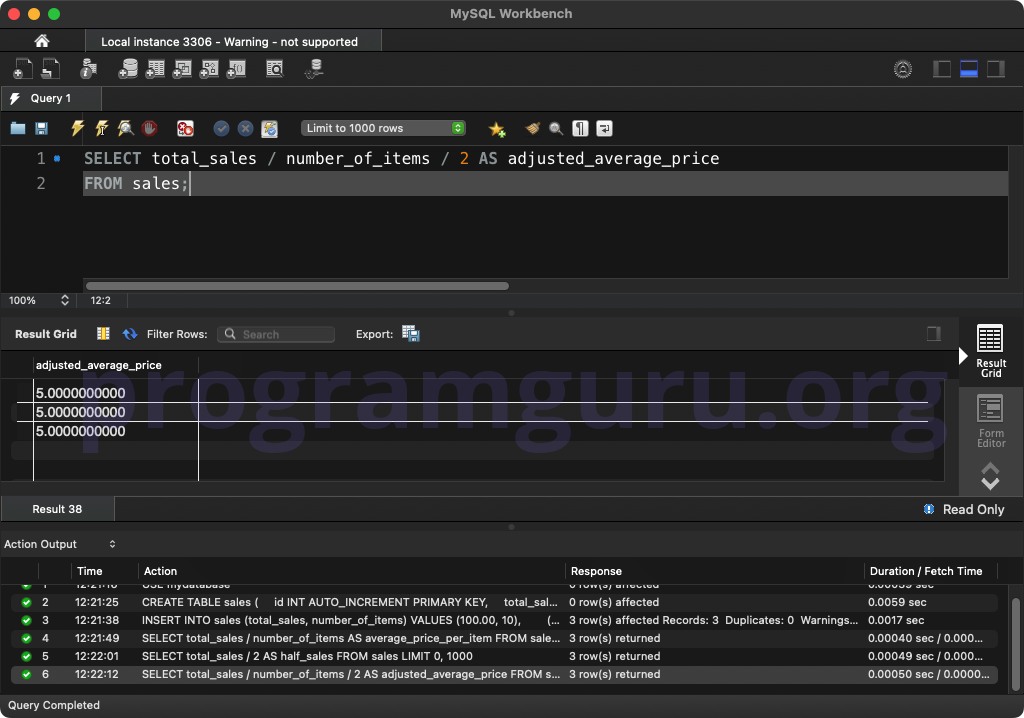
Conclusion
The MySQL /
operator is a powerful tool for performing arithmetic calculations in SQL queries. Understanding how to use the /
operator is essential for effective data manipulation and analysis in MySQL.