MySQL INSERT() String Function
MySQL INSERT() String Function
The MySQL INSERT()
string function inserts a substring at a specified position within a string and can optionally replace a specified number of characters. This function is essential for modifying strings by inserting new substrings in SQL queries.
Syntax
SELECT INSERT(original_string, position, length, new_substring) AS result
FROM table_name;
The INSERT()
function has the following components:
original_string
: The original string to be modified.position
: The position in the original string where the insertion should start.length
: The number of characters in the original string to be replaced.new_substring
: The substring to be inserted into the original string.result
: An alias for the resulting modified string.table_name
: The name of the table from which to retrieve the data.
Example MySQL INSERT() String Function
Let's look at some examples of the MySQL INSERT()
string function:
Step 1: Using the Database
USE mydatabase;
This query sets the context to the database named mydatabase
.
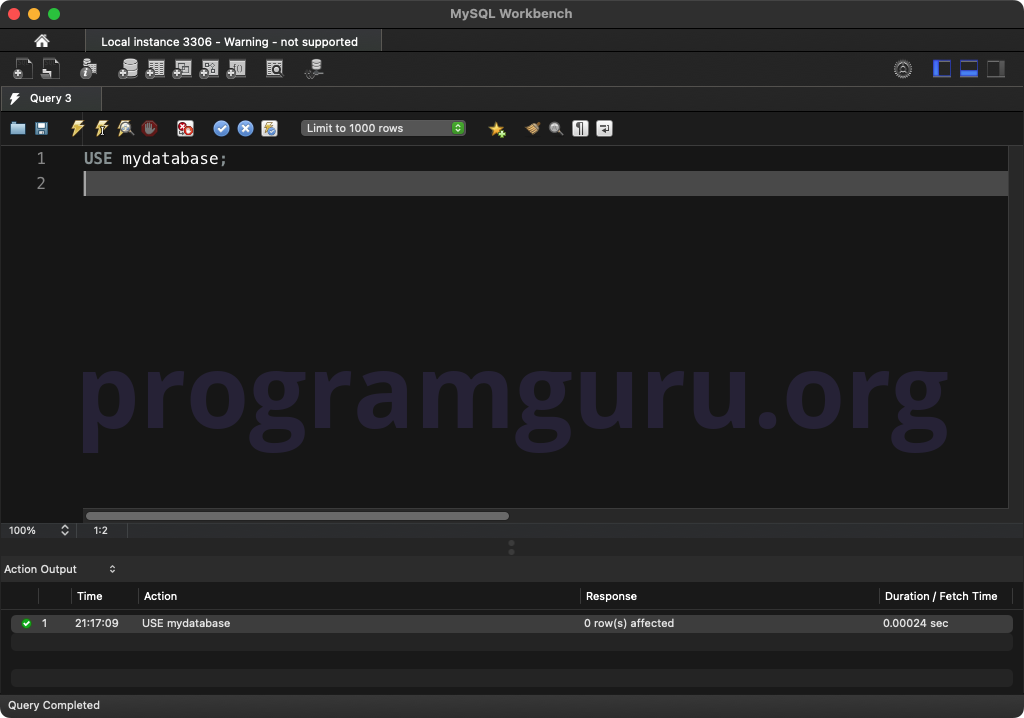
Step 2: Creating a Table
Create a table to work with:
CREATE TABLE sample_strings (
id INT AUTO_INCREMENT PRIMARY KEY,
value VARCHAR(255) NOT NULL
);
This query creates a table named sample_strings
with columns for id
and value
.
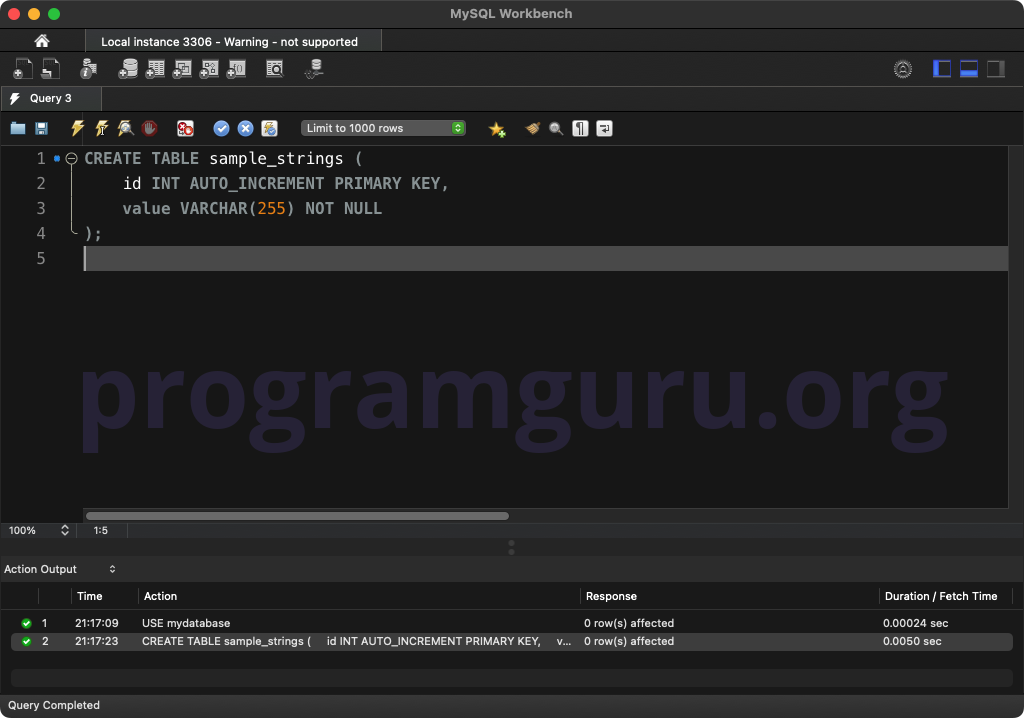
Step 3: Inserting Initial Rows
Insert some initial rows into the table:
INSERT INTO sample_strings (value)
VALUES ('Hello world'),
('MySQL database'),
('String function'),
('Insert example'),
('Test case');
This query inserts five rows into the sample_strings
table.
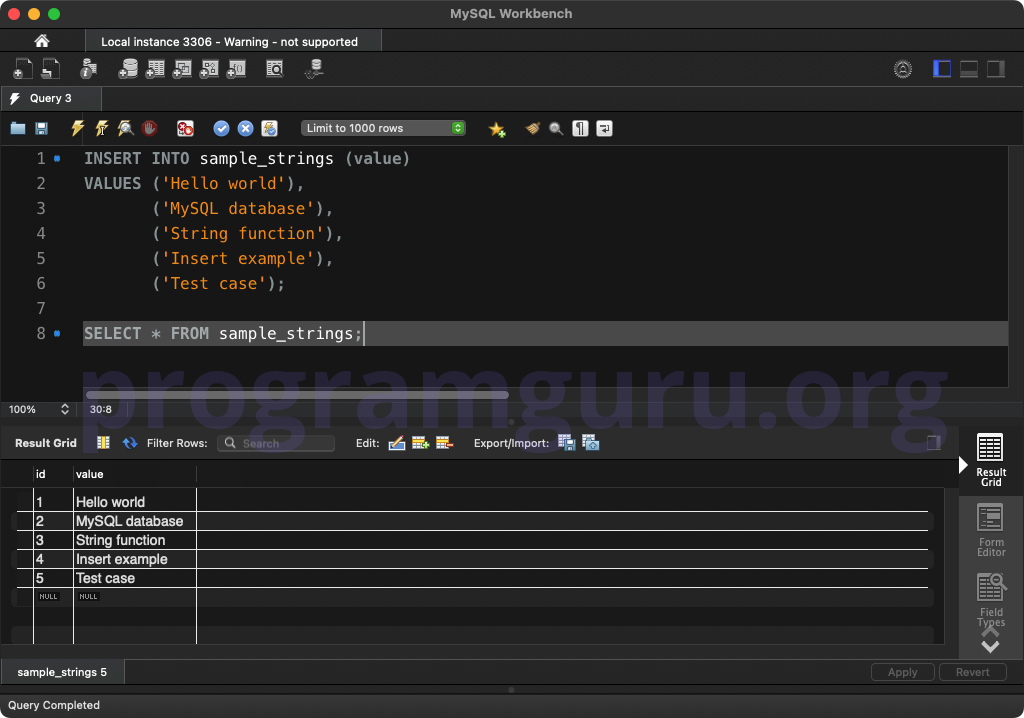
Step 4: Using INSERT() with WHERE Clause
Use the INSERT()
function to modify a string by inserting a new substring:
SELECT value, INSERT(value, 7, 5, 'planet') AS modified_value
FROM sample_strings;
This query retrieves the value
column from the sample_strings
table and returns the modified string where 'planet' is inserted at position 7, replacing 5 characters.
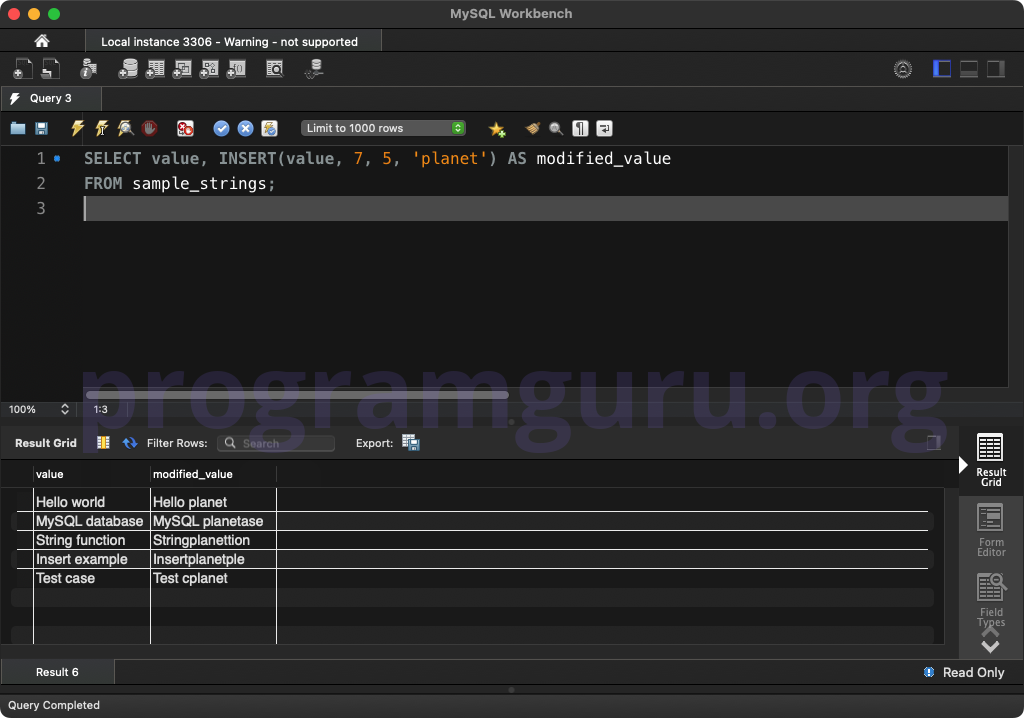
Step 5: Using INSERT() with Multiple Columns
Use the INSERT()
function with multiple columns:
SELECT id, value, INSERT(value, 4, 0, 'SQL ') AS modified_value
FROM sample_strings;
This query retrieves the id
and value
columns from the sample_strings
table and returns the modified string where 'SQL ' is inserted at position 4 without replacing any characters.
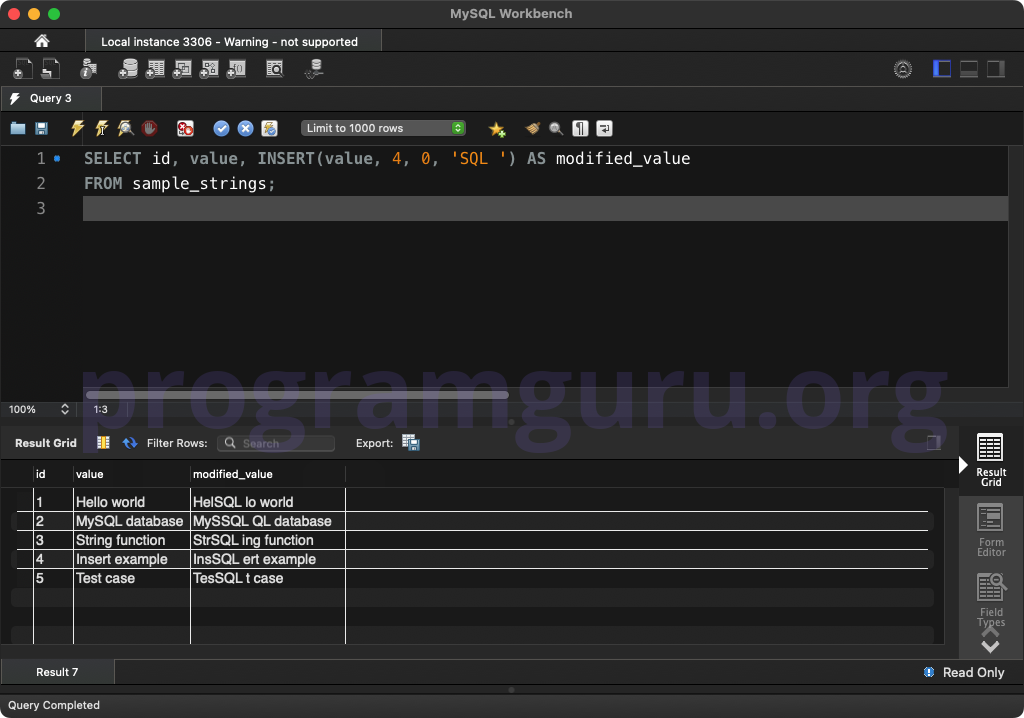
Step 6: Using INSERT() with Constants
Use the INSERT()
function with constants:
SELECT INSERT('Sample text', 8, 4, 'data') AS modified_constant;
This query modifies the constant string 'Sample text' by inserting 'data' at position 8, replacing 4 characters.
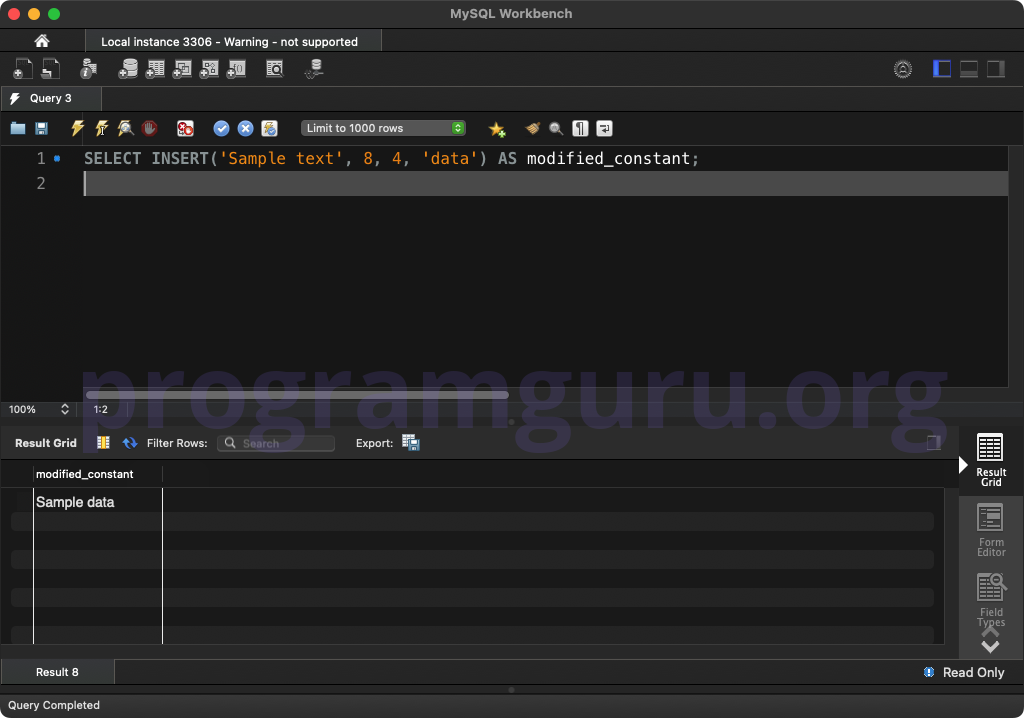
Conclusion
The MySQL INSERT()
function is a powerful tool for modifying strings by inserting new substrings in SQL queries. Understanding how to use the INSERT()
function is essential for effective data querying and manipulation in MySQL.