Check if Directory is Empty in Python
Python - Check if directory is empty
To check if a directory is empty or not in Python, you can use the os.listdir
function. The function returns a list of files and sub-directories in the directory. If this list is empty, then the directory is empty.
Empty directory should have no files or sub-directories.
The syntax of the boolean expression to check if the directory is empty is
not os.listdir('path/to/directory')
The above expression returns a boolean value of True if the directory is empty, or False if the directory is not empty.
Note: The 'path/to/directory'
in the above expression should be replaced with the actual path of the directory you want to check.
Example
In the following program, we check programmatically if the directory myfolder/resources
is empty or not.
Directory
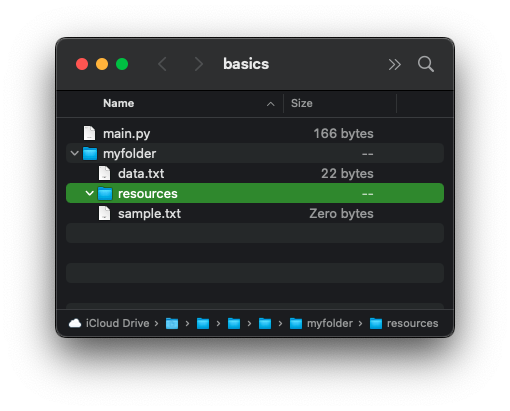
We use the previously mentioned boolean expression, to check if the directory is empty or not, as a condition in the if-else statement.
Python Program
import os
path_to_directory = "myfolder/resources"
if not os.listdir(path_to_directory):
print("Directory is empty.")
else:
print("Directory is not empty.")
Output
Directory is empty.
Faster Way
If you are using Python3.5 or higher, there is a method whose performance is better than the above mentioned solution.
os.scandir('path/to/directory')
returns an iterator to the files and sub-directories in the given directory. To check if the directory is empty, all we have to do is if there is no any item in this iterator.
In the following example, we use os.scandir()
to check if the given directory is empty or not.
Python Program
import os
path_to_directory = "myfolder/resources"
if not any(os.scandir(path_to_directory)):
print("Directory is empty.")
else:
print("Directory is not empty.")
Output
Directory is empty.
Summary
In this tutorial of Python Examples, we learned how to check if a directory is empty or not by checking if there are any files or sub-directories in the specified directory.