Rename Directory in Python
Python - Rename Directory
To rename directory in Python, import os library, call os.rename() function, and pass old directory path and new directory path as arguments.
The syntax to call os.rename() function to rename a directory is
import os
os.rename('current_dir_path', 'new_dir_path')
Example
In the following program, we will rename the directory "myfolder" to "myfolder_1".
Original Directory
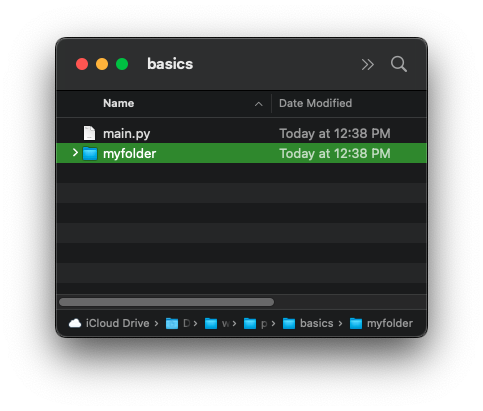
Python Program
import os
current_dir_name = 'old_directory_name'
new_dir_name = 'new_directory_name'
try :
os.rename(current_dir_name, new_dir_name)
print('Directory renamed successfully.')
except FileNotFoundError:
print('Could not rename directory.')
print('Directory not found.')
except PermissionError:
print('Could not rename directory.')
print('Issue with permission.')
Output
Directory renamed successfully.
Renamed Directory
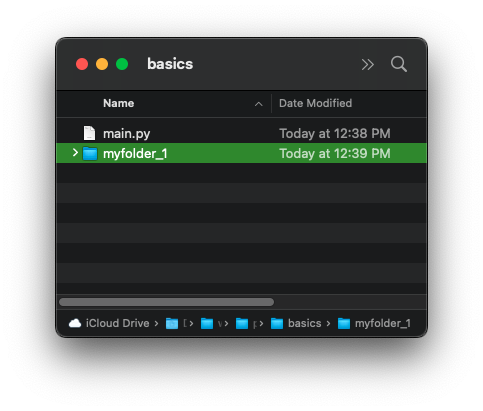
Summary
In this tutorial of Python Examples, we learned how to rename a directory using os.rename() function, with the help of examples.