Open a File in Read Mode in Python
To open a file in read mode in Python, call open() function and pass the file path for first argument, and “r” for the second argument. The second argument is the mode in which open() function opens given file, and “r” specifies the read mode.
The syntax to open a file in read mode is
open(file_path, "r")
where file_path is the path to the file which we would like to open.
When a file is opened in read mode, we can only read the contents of the file. We cannot modify the contents of the file, in any way, like removing the contents, updating the contents, or appending to content.
Once the file is opened in read mode, you can read the contents of the file as a string, or as a list of lines, or line by line in a loop, etc.
Examples
1. Open file in read mode and print its content
In the following program, we shall open a file named “data.txt” in read mode, and print the content of this file to standard output.
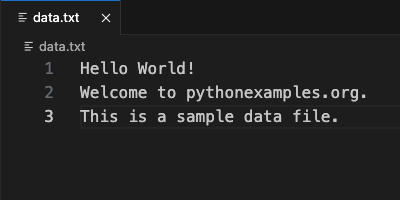
Python Program
file_path = "data.txt"
with open(file_path, "r") as file:
content = file.read()
print(content)
Output
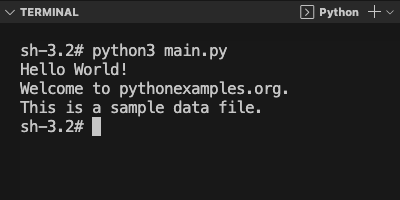
Summary
In this tutorial of Python File Operations, we have seen how to open a file in read mode in Python using open() built-in function, with examples.