Python – Get File Modified Time
To get file modified timestamp in Python, you can use the os.path
module. Call os.path.getmtime()
function and pass the file path as argument to the function.
The function returns a number giving the number of seconds since the epoch. You may use datetime library to format it to a specific datetime format.
The syntax to call os.path.getmtime() function to get the time when the file was modified is
import os
os.path.getmtime(file_path)
Example
In the following program, we get the modified time of the file data.txt
programmatically using os.path.getmtime()
function.
File
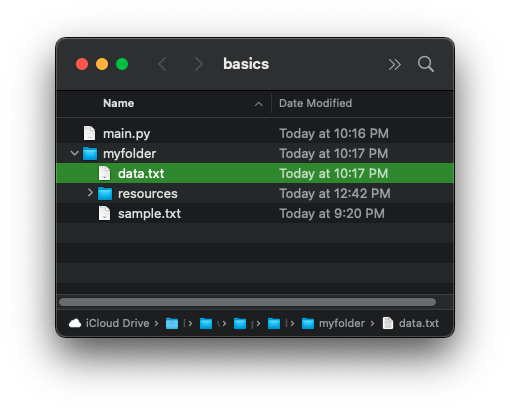
Python Program
import os
import datetime
file_path = 'myfolder/data.txt'
#get modified time
n = os.path.getmtime(file_path)
#convert epoch to a timestamp
timestamp = datetime.datetime.fromtimestamp(n).strftime('%c')
print(timestamp)
Output
Mon Jan 16 22:17:35 2023
Summary
In this tutorial of Python Examples, we learned how to get the modified time of a file using os.path.getmtime() function, with the help of examples.