Contents
Python Create Directory
To create a directory using Python program, use os.mkdir() function and pass directory path to be created as argument to the function.
In this tutorial, we shall learn how to create a directory, with the help of example programs.
Syntax of os.mkdir()
The syntax of python os.mkdir() function is:
os.mkdir(path, mode=0o777, *, dir_fd=None)
where path is the directory location to be created and mode is the file permissions to be assigned while creating the directory.
Examples
1. Create a Directory or Folder
In this example, we will create a directory named “sample”.
Output
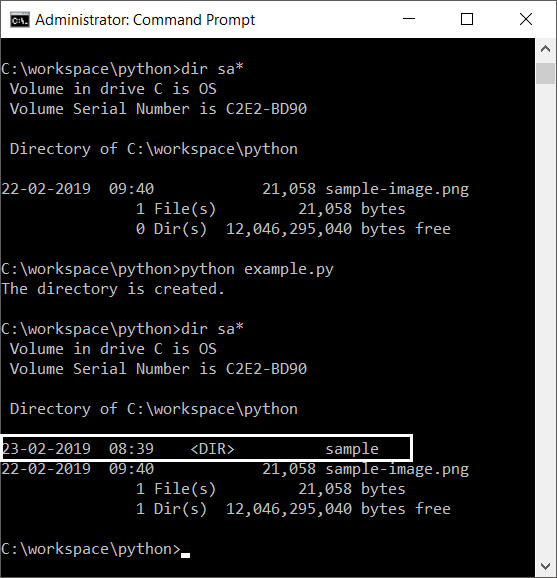
If you provide the the directory name alone, the directory will be created at the path from which you are running the python program.
If you would like to create the directory at a specific location, provide the complete or absolute path of the directory.
2. Create Directory with the name that is already present
If you try to create a directory that is already present, you will get FileExistsError.
Output
Traceback (most recent call last):
File "example.py", line 3, in <module>
os.mkdir('sample')
FileExistsError: [WinError 183] Cannot create a file when that file already exists: 'sample'
The error clearly states that ‘Cannot create a file when that file already exists’. The stack trace also provides line of error in the python file and file that it tried to create.
You can check if the directory is already present, and then create the directory.
Python Program
import os
dirPath = 'C:/workspace/python/sample'
if not os.path.isdir(dirPath):
print('The directory is not present. Creating a new one..')
os.mkdir(dirPath)
else:
print('The directory is present.')
CopySummary
In this tutorial of Python Examples, we learned how to create a new directory, with the help of example Python programs.