Python – Write list of strings to text file
To write a list of strings to a text file in Python, we can use writelines() method. Get the file object opened in write mode for given file path, and then call writelines() method on the file object.
The writelines() method takes an iterable of strings as argument, and writes the lines to the file.
Example
In the following example, we will take a list of strings and write this list of strings as lines in the text file located at myfolder/sample.txt
path.
Python Program
import os
path_to_file = "myfolder/sample.txt"
lines = [
'Apple is red.',
'Guava is green.',
'Mango is yellow.'
]
with open(path_to_file, 'w') as file:
file.writelines(line+'\n' for line in lines)
print('Lines are written to file.')
Output
Lines are written to file.
with statement takes care of closing the file.
sample.txt
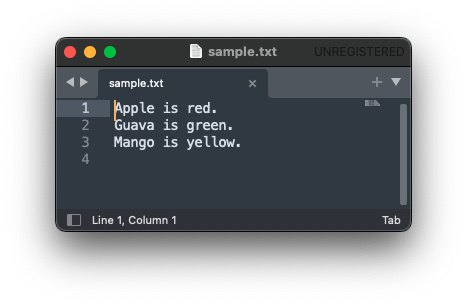
Note that this kind of writing to text file, overwrites the existing data in file.
Summary
In this tutorial of Python Examples, we learned how to write a list of strings to a text file using writelines() function, with the help of example programs.