Python - Write to File
Write to File in Python
In this tutorial, you will learn how to write content to a file in Python, with examples.
To write content to a file in Python
- Open the file in write mode using open() built-in function
- Call the write() method on the file instance returned by open() function, and pass the content as argument to the write() method.
- You may write the whole content in a single go, or write each line to the file.
There are also other ways of writing content to a file in Python. It is by using print() builtin function.
Please note that if the specified file already exists, then the existing content of the file is overwritten with the given new content.
Now, let us go through some examples, covering the above said ways of writing to a file in Python.
Examples
1. Write string to file in Python
In the following program, we shall open a file named "data.txt" in write mode, and write the text in a string variable to the file, using write() method.
Python Program
file_path = "data.txt"
content = "Hello World! This is some sample text."
with open(file_path, "w") as file:
file.write(content)
Output
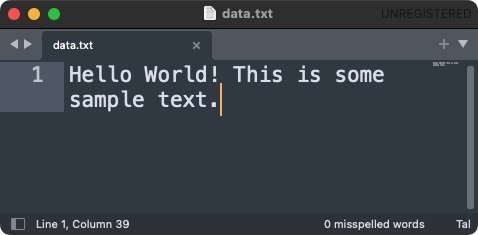
2. Write list of strings as lines to a file in Python
In the following program, we shall open a file named "data.txt" in write mode, and write each of the string in a given list of strings, as a line in the file.
When we write a line to the file, we append a new line character to each line.
Python Program
file_path = "data.txt"
my_list = [
"This is first line.",
"This is line 2.",
"This is last line.",
]
with open(file_path, "w") as file:
for line in my_list:
file.write(line + "\n")
Output
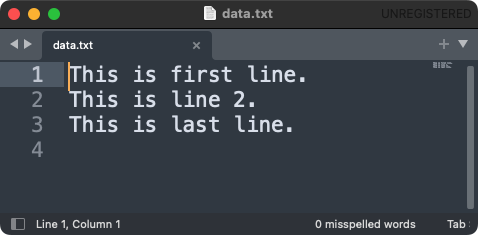
3. Write lines to file using writelines() method in Python
In the following program, we shall open a file named "data.txt" in write mode, and write given list of strings, as lines in the file, using writelines() method of the file instance.
Please observe the list in the program. New line character has been given at the end of each string in the list. If the list does not have trailing new lines for the string items, you may want to add a new line as we did in the previous example.
Python Program
file_path = "data.txt"
my_list = [
"This is first line.\n",
"This is line 2.\n",
"This is last line.",
]
with open(file_path, "w") as file:
file.writelines(my_list)
Output
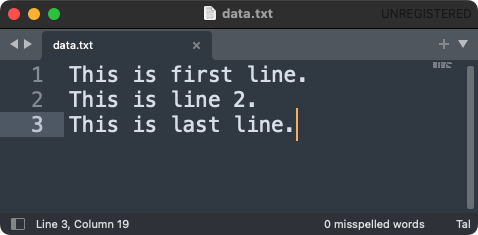
4. Write to file using print() function in Python
In the following program, we shall write content to a file using print() function.
By default, print() function prints given data to standard output. But if specify a file stream to the print() function using file parameter, the print() function prints the given content to the specified file.
Python Program
file_path = "data.txt"
content = "Hello World! This is some sample text."
with open(file_path, "w") as file:
print(content, file=file)
Output
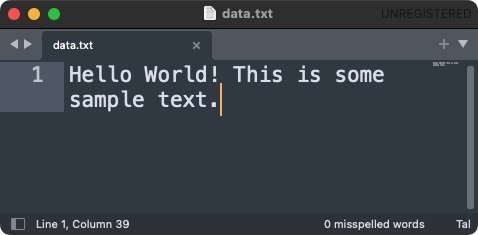
Summary
In this tutorial of Python File Operations, we have seen how to write to a file in Python, with the help of example programs.