Python - Print to File
Print to File in Python
To print data or text to a file in Python,
- Open file in append mode using open() built-in function.
- Call print statement and pass the data as first argument, and the file as named parameter.
- The data passed to the print() statement shall be written to the file.
The syntax to open a file in append mode is
f = open('my_log.txt', 'a')
The syntax to print a string to the file is
print("Hello World", file=f)
The syntax to print a list to the file is
print(['apple', 'banana'], file=f)
Similarly we can print other data types to a file as well.
Examples
1. Print string data to file in Python
In the following program, we shall open a file named "my_log.txt" in append mode.
We shall take a string value in text variable, and pass it as argument to the print() function. Also, pass the file for the file named parameter to the print() function.
Python Program
f = open('my_log.txt', 'a')
# Print string to file
text = "Hello World! Welcome to new world."
print(text, file=f)
Output
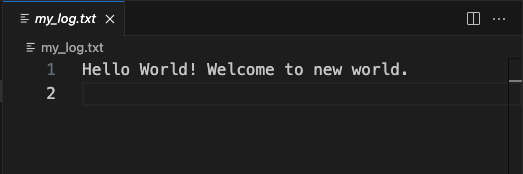
The string value is printed to the file.
If the file is already present, our data is appended to the existing data in the file.
If the file is not present, then a new file would be created.
2. Print list data to file in Python
In the following program, we shall print list data to file in Python. We shall print to the same file, that we used in the previous example.
Python Program
f = open('my_log.txt', 'a')
# Print list to file
print(['apple', 'banana', 'cherry'], file=f)
Output
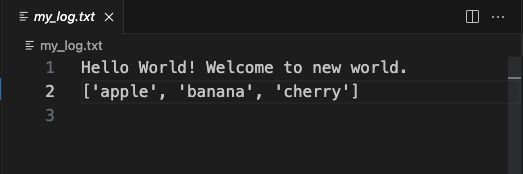
The list data is printed to the file.
3. Print multiple data to file in Python
In the following program, we shall use multiple print statements to print different types of data to file in Python. We shall delete the file created from the previous example, and run the program.
Python Program
f = open('my_log.txt', 'a')
# Print string to file
text = "Hello World! Welcome to new world."
print(text, file=f)
# Print list to file
print(['apple', 'banana', 'cherry'], file=f)
# Print tuple to file
print(('apple', 25), file=f)
# Print set to file
print({'a', 'e', 'i', 'o', 'u'}, file=f)
# Print dictionary to file
print({'mac' : 25, 'sony' : 22}, file=f)
Output
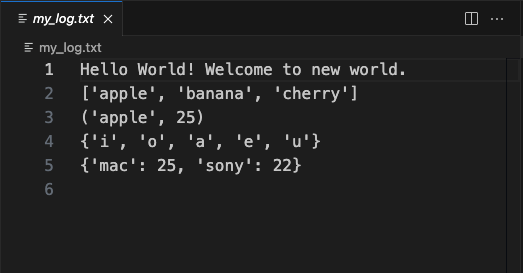
Summary
In this tutorial of Python File Operations, we have seen how to print data to a file in Python using print() built-in function, with examples.