Python – Get File Created Time
To get file created timestamp in Python, you can use the os.path
module. Call os.path.getctime()
function and pass the file path as argument to the function.
The function returns a number giving the number of seconds since the epoch. You may use datetime library to format it to a specific datetime format.
The syntax to call os.path.getctime() function to get the time when the file was created is
import os
os.path.getctime(file_path)
Note: The function returns the system’s ctime (created time) which, on some systems (like Unix) is the time of the last metadata change, and, on others (like Windows), is the creation time.
Example
In the following program, we will get the created time of the file data.txt
programmatically using os.path.getctime()
function.
File
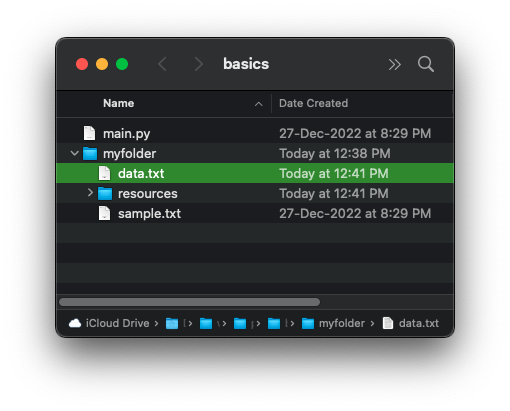
Python Program
import os
import datetime
file_path = 'myfolder/data.txt'
#get created time
n = os.path.getctime(file_path)
#convert epoch to a timestamp
timestamp = datetime.datetime.fromtimestamp(n).strftime('%c')
print(timestamp)
Output
Mon Jan 16 12:41:44 2023
Summary
In this tutorial of Python Examples, we learned how to get the created time of a file using os.path.getctime() function, with the help of examples.