Python – Check if file exists
To check if a file exists in Python, you can use the os.path
library. Import os library, call the os.path.exists()
function and pass the path to file as argument to the function.
The syntax to call os.path.exists() function to check if a file exists
import os
os.path.exists('path/to/file')
The function exists() returns a boolean value of True if the file exists, or False if the file does not exist.
Example
In the following program, we check programmatically if the file data.txt
exists or not using os.path.exists()
function.
File
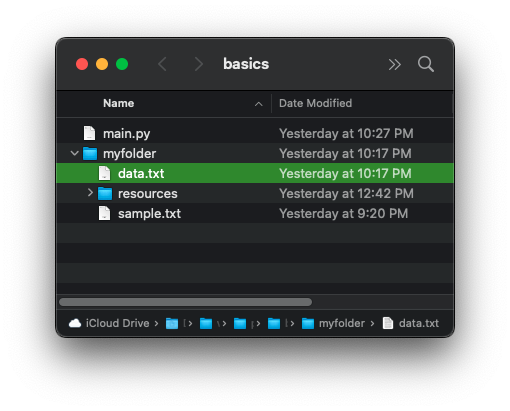
We use the function call os.path.exists()
as a condition in the if-else statement.
Python Program
import os
path_to_file = 'myfolder/data.txt'
if os.path.exists(path_to_file):
print("File exists.")
else:
print("File does not exist.")
Output
File exists.
Summary
In this tutorial of Python Examples, we learned how to check if a file exists or not using os.path.exists() function, with the help of examples.