Python – Get File Extension
To get file extension in Python, you can use the os.path module to get the extension of a file. Call os.path.splitext() function and pass the file path as argument to the function. The function returns the root, and extension as a tuple.
The syntax to call os.path.splitext() function to get the extension of a file at file_path location is
import os
_, extension = os.path.splitext(file_path)
Example
In the following program, we will get the extension of the file data.txt programmatically using os.path.splitext() function.
File
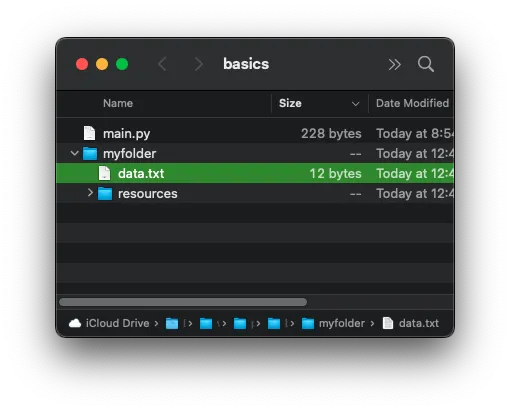
Python Program
import os
file_path = 'myfolder/data.txt'
_, file_extension = os.path.splitext(file_path)
print(file_extension)
CopyOutput
.txt
If there is no extension to the file, then splitext() returns an empty string, as shown in the following example.
Python Program
import os
file_path = 'myfolder/data'
_, file_extension = os.path.splitext(file_path)
print(file_extension)
CopyOutput
Summary
In this tutorial of Python Examples, we learned how to get the extension of a file using os.path.splitext() function, with the help of examples.