Python – Open file in write mode
To open a file in write mode in Python, call open() function and pass the file path for first argument, and “w” for the second argument. The second argument is the mode in which open() function opens given file, and “w” specifies the write mode.
The syntax to open a file in write mode is
open(file_path, "w")
where file_path is the path to the file which we would like to open.
When a file is opened in write mode, we can write content to the file. If the file is not already present, then a new file is created. If the file is already present, then existing content shall be overwritten.
Examples
1. Open file in write mode in Python, given file is not present
In this example, we have to open a file at path “data.txt” in write mode, and write given text content to the file.
Python Program
# Given file path
file_path = "data.txt"
# Text content
content = "Hello World!"
# Open file in write mode
with open(file_path, "w") as file:
# And write content to the file
file.write(content)
print("Content written to file.")
The file specified by the given path “data.txt” is not present. Therefore, a new file would be created at the specified path. Since, the path is relative, data.txt would be created from where the python file is run.
The screenshot of the Visual Studio Code editor, with Python file is given below.
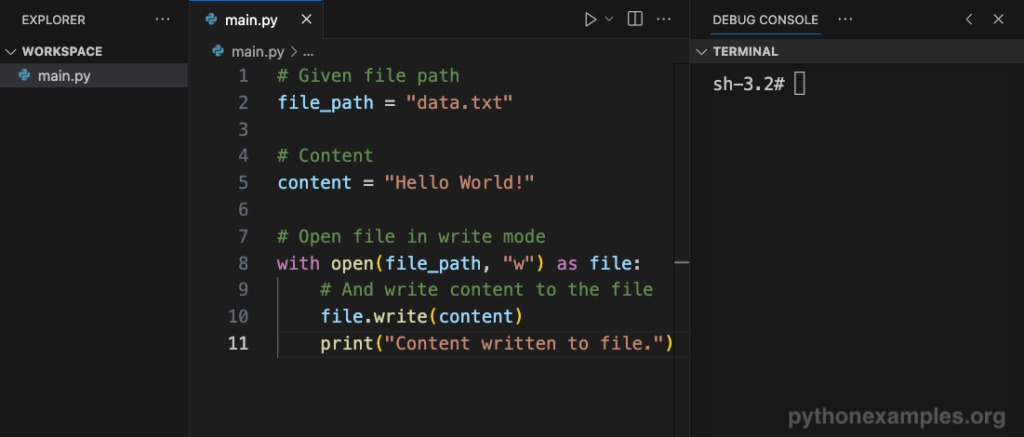
If you observe under the WORKSPACE, there is no data.txt file.
Now, the below screenshot is after running this Python program.
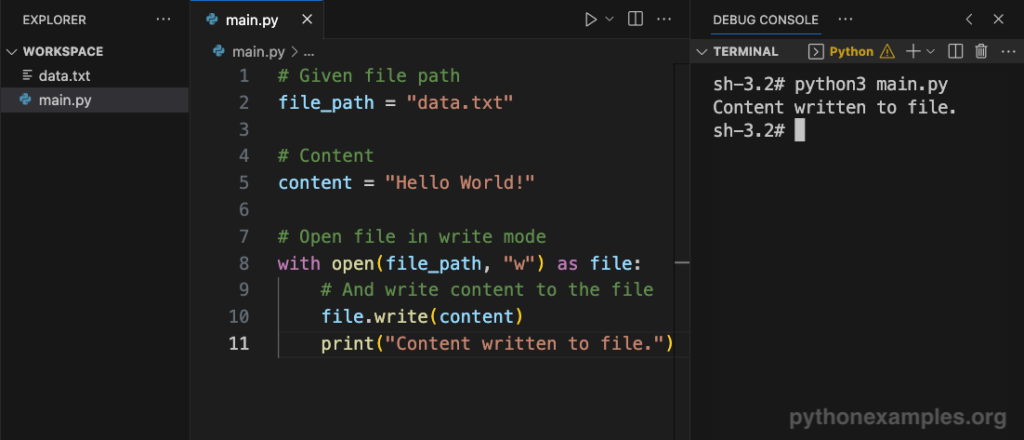
A new file data.txt is created next to main.py file.
Let us open the data.txt file.
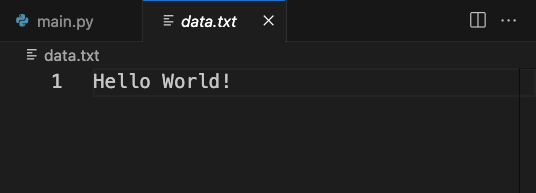
The specified content is written to the file.
2. Open file in write mode in Python, given file is already present
In this example, we have to open a file named “data.txt” in write mode, and write give text content to the file.
Python Program
# Given file path
file_path = "data.txt"
# Content
content = "Welcome to pythonexamples.org"
# Open file in write mode
with open(file_path, "w") as file:
# And write content to the file
file.write(content)
print("Content written to file.")
The file specified by the given path “data.txt” is already present, because we have created the file in our previous example. Therefore, when we try to open this existing file in write mode, then the original content in the file is overwritten with the new content.
The screenshot of the Visual Studio Code editor, with Python file and data.txt is given below.
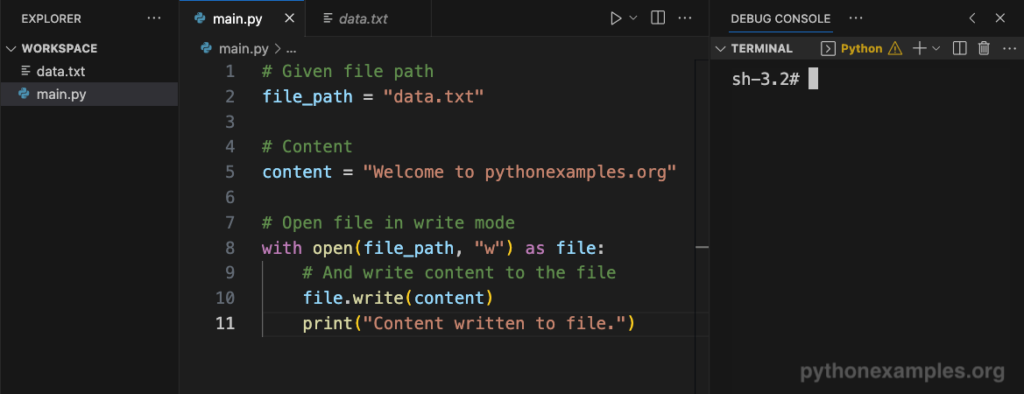
Run the program and see the contents of data.txt.
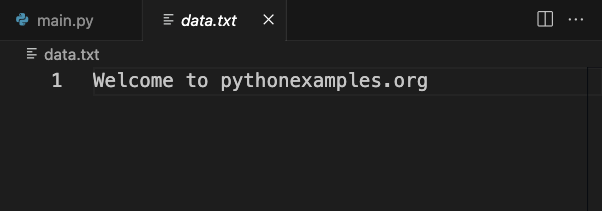
The original contents have been overwritten.
Summary
In this tutorial of Python File Operations, we have seen how to open a file in write mode in Python using open() built-in function. We have gone through examples of how to open a file in write mode when the given file is not already present, and write content to the file, and also when the given file is already present.