Check if File is Readable in Python
Python - Check if file is readable
To check if a file is readable or not in Python, you can use the os
library. Import os library, call the os.access() function and pass the file path and mode (os.R_OK
, to check if file is readable) as arguments.
The syntax to call os.access() function to check if a file is readable is
os.access('path/to/file', os.R_OK) == 0
The function call returns a boolean value of True if the file is readable, or False if the file is not readable.
Note: The 'path/to/file'
in the above expression should be replaced with the actual path of the file, including the file name, you want to check.
Example
In the following program, we check programmatically if the file myfolder/sample.txt
is readable or not.
File
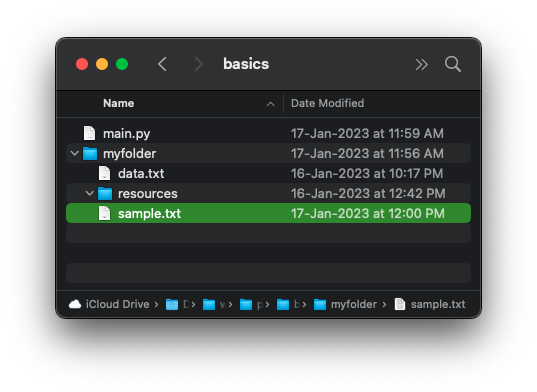
Python Program
import os
path_to_file = "myfolder/sample.txt"
if os.access(path_to_file, os.R_OK):
print("File is readable.")
else:
print("File is not readable.")
Output
File is readable.
Summary
In this tutorial of Python Examples, we learned how to check if a file is readable or not by using os.access() function.