Contents
Check if Given Path is File or Directory
When you get a string value for a path, you can check if the path represents a file or a directory using Python programming.
To check if the path you have is a file or directory, import os module and use isfile() method to check if it is a file, and isdir() method to check if it is a directory.
In this tutorial, we shall learn how to check if a given path is file or folder, with the help of well detailed examples.
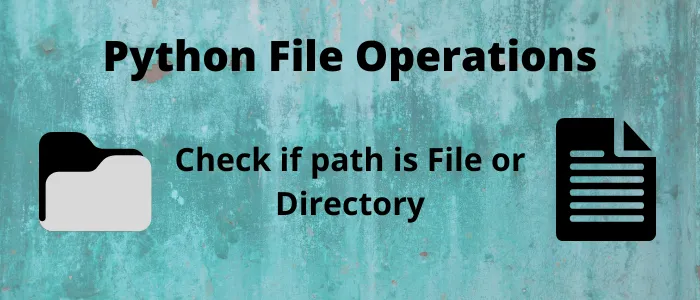
Sample Code
Following is a quick sample code snippet that demonstrates the usage of isfile() and isdir() functions.
import os
# Checks if path is a file
isFile = os.path.isfile(fpath)
# Checks if path is a directory
isDirectory = os.path.isdir(fpath)
Both the functions return a boolean value if the specified file path is a file or not; or directory or not.
Examples
1. Check if the given path is a File
In this example, consider that we have a file specified by the variable fpath. We will use isfile() method to check if we can find out if it is a file or not.
Python Program
import os
fpath = 'D:/workspace/python/samplefile.txt'
isFile = os.path.isfile(fpath)
print('The file present at the path is a regular file:', isFile)
Output
The file present at the path is a regular file: True
Now let us try with a path, that is a folder, passed as argument to isfile().
Python Program
import os
fpath = 'D:/workspace/python/'
isFile = os.path.isfile(fpath)
print('The file present at the path is a regular file:', isFile)
Output
The file present at the path is a regular file: False
That’s good. We are able to recognize if the specified path is a file or not.
2. Check if given path is a Directory
In the following example, consider that we have a folder or directory specified by the variable fpath. We will use isdir() method to check if we can find out if it is a file.
Python Program
import os
fpath = 'D:/workspace/python/'
isDirectory = os.path.isdir(fpath)
print('Path points to a Directory:', isDirectory)
Output
Path points to a Directory: True
Now let us try with a path, that is a file, passed as argument to isdir().
Python Program
import os
fpath = 'D:/workspace/python/samplefile.txt'
isDirectory = os.path.isdir(fpath)
print('Path points to a Directory:', isDirectory)
Output
Path points to a Directory: False
Again that’s good. It recognized if the path provided is a directory or not.
Summary
In this tutorial of Python Examples, we learned how to check if a given path is file or directory in Python, using isfile() and isdir(), with the help of well detailed examples.