Python - Print from File
Print from File in Python
In this tutorial, you will learn how to print from a file in Python, with examples.
To print from a file in Python, open the file in read mode using open() built-in function, and read the whole content of the file using read() method of file instance, or read the file line by line by using the file instance as iterator in a For loop.
Examples
1. Print content of file
In the following program, we shall open a file named "data.txt" in read mode, and print the whole content of this file to standard output.
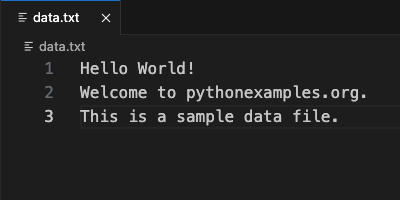
Python Program
file_path = "data.txt"
with open(file_path, "r") as file:
content = file.read()
print(content)
Output
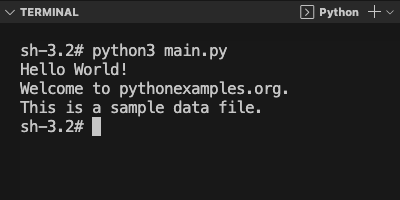
2. Print content of file line by line
In the following program, we shall open a file named "data.txt" in read mode, and print the content of this file line by line to standard output using a For loop.
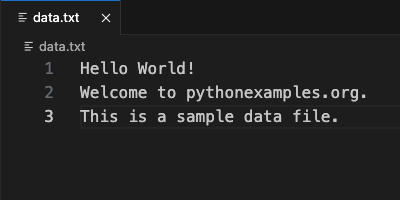
Python Program
file_path = "data.txt"
with open(file_path, "r") as file:
i = 0
for line in file:
i += 1
print(f"Line {i} : {line.strip()}")
Output
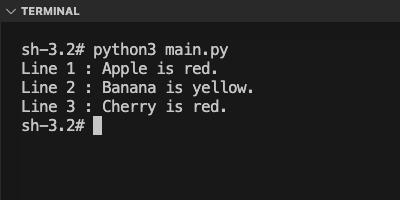
Summary
In this tutorial of Python File Operations, we have seen how to print from a file in Python using open() built-in function, with examples.