How to Write String to Text File in Python?
Python - Write String to Text File
You can save or write string to a text file in disk storage using Python.
To write string to a Text File, follow these steps.
- Open file in write mode using open() function. Call open() function and pass the path to text file as first argument, and the write mode "w" as second argument. The method returns a file object.
- Write string to the file stream using write() method. In the previous step we got a file object. Call write() method on this file object, and pass the text content string as argument. The method writes the string to text file, and returns an integer value specifying the number of characters written to text file.
- Close the file using close() method. Call close() method on the file object.
Examples
In the following examples, we shall demonstrate how to write a given string to a text file, by explaining the code in detail.
1. Write string to text file in Python
In this example, we have to write a string "Hello World"
to a text file at given file path sample.txt.
Steps
Let us follow the steps that we already mentioned, to write the given string to the specified file.
- Call open() method and pass the file path
"sample.txt"
as first argument and"w"
as second argument. open() method opens the file in write mode. The method returns a file object. Store the file object in a variable, say text_file.
text_file = open("sample.txt", "w")
- Given file content in a variable, content.
content = "Hello World"
- Call write() method on the file object text_file, and pass content as argument. The write() method writes given string to the file stream, and returns the number of character written. Based on the number of characters written, we can make sure whether given content is written to the file successfully, or not.
n = text_file.write(content)
- Call close() method on the file object text_file. The close() method closes the file stream.
text_file.close()
Program
The complete program to write "Hello World" string to sample.txt text file is given below.
Python Program
# Open text file in write mode
text_file = open("sample.txt", "w")
# Take content
content = "Hello World"
# Write content to file
n = text_file.write(content)
if n == len(content):
print("Success! String written to text file.")
else:
print("Failure! String not written to text file.")
# Close file
text_file.close()
Output
Success! String written to text file.
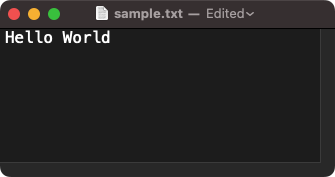
Please note that this approach overwrites the data, if the file is already present. But, if the file is not present, it creates a new file, and then writes the given string to specified file path.
2. Write string to text file in text mode in Python
A file can be opened in two modes: the first one is text mode, and the second one is binary mode. If you do not specify text or binary in the mode, then the file is by default opened in text mode, which is what happened in the previous example. However, you can explicitly specify the text mode by writing "t" (t for text) next to the "w" (w for write) for the mode argument.
open("sample.txt", "wt")
In the following example, we shall explicitly open the file in write text mode and write the string to specified text file.
Python Program
# Open text file in write+text mode
text_file = open("sample.txt", "wt")
# Take content
content = "Hello World"
# Write content to file
n = text_file.write(content)
if n == len(content):
print("Success! String written to text file.")
else:
print("Failure! String not written to text file.")
# Close file
text_file.close()
Output
Success! String written to text file.
Writing other than String to Text File
If you would like to write any Python object other than string or a bytes object to a file, using write() method, you should first convert that Python object to a string or bytes object, and then write the resulting string to the text file.
Summary
In this tutorial, we learned how to write a string to a text file, with the help of example programs. We have taken a sample text in a string variable, and then used open() and file.write() to write the string content to the file.