Python - Get list of files in directory
Get list of files in directory in Python
To get the list of files in a directory in Python,
- Import listdir from os, and import isfile, and join from os.path.
- Given a directory path.
- Get the list of files and directories in the given directory path using
listdir(dir_path)
. - For each item in the list returned by
listdir(dir_path)
, check if the item is a file or directory. Consider the item if it is a file, or discard the item if it is not a file. You can check if item is a file or not usingisfile()
method.
If dir_path is the given directory path, then the statement to get the list of files at the directory path is
files = [file for file in listdir(dir_path) if isfile(join(dir_path, file))]
Examples
1. Get list of files in directory in Python
In the following program, we shall get the list of only files at the given directory path "data/directory1", and print the list of files to standard output.
Python Program
from os import listdir
from os.path import isfile, join
dir_path = "data/directory1"
files = [file for file in listdir(dir_path) if isfile(join(dir_path, file))]
for file in files:
print(file)
Output
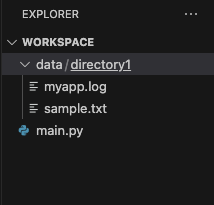
Summary
In this tutorial of Python File Operations, we have seen how to get the list of files in a given directory path, with examples.