Python – Check if file is empty
To check if a file is empty or not in Python, you can use the os.path
library. Import os library, get the file size using os.path.getsize()
function and check if the size is zero or not.
Empty file must have a size of zero bytes.
The syntax of the boolean expression to check if the file is empty is
os.path.getsize('path/to/file') == 0
The above expression returns a boolean value of True if the file is empty, or False if the file is not empty.
Note: The 'path/to/file'
in the above expression should be replaced with the actual path of the file you want to check.
Example
In the following program, we check programmatically if the file myfolder/sample.txt
is empty or not.
File
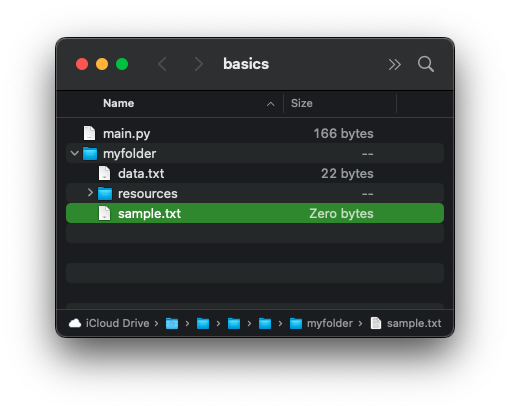
We use the above boolean expression, to check if the file is empty or not, as a condition in the if-else statement.
Python Program
import os
path_to_file = "myfolder/sample.txt"
if os.path.getsize(path_to_file) == 0:
print("File is empty.")
else:
print("File is not empty.")
Output
File is empty.
Summary
In this tutorial of Python Examples, we learned how to check if a file is empty or not by checking if the file size is zero or not.