Python - Create Directory - os.mkdir()
Python Create Directory
To create a directory using Python program, use os.mkdir() function and pass the directory path to be created as an argument to the function.
In this tutorial, we shall learn how to create a directory, with the help of example programs.
Syntax of os.mkdir()
The syntax of Python os.mkdir() function is:
os.mkdir(path, mode=0o777, *, dir_fd=None)
Here:
- path: The location where the new directory is to be created.
- mode: (Optional) Specifies the file permissions (default:
0o777
, which means read, write, and execute permissions for all users).
Examples
1. Create a Directory or Folder
In this example, we will create a directory named "sample".
Python Program
import os
os.mkdir('sample')
print('The directory is created.')
Output
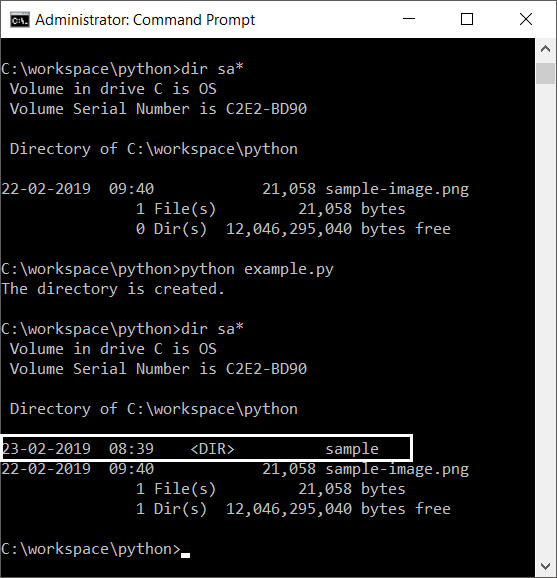
Explanation (Step-by-Step):
- Import the
os
module, which provides functions to interact with the file system. - Call
os.mkdir('sample')
to create a new directory namedsample
in the current working directory. - If the operation is successful, the directory will be created.
- Print the message
'The directory is created.'
to confirm the action.
If you provide only the directory name, the directory will be created in the current working directory. To create the directory at a specific location, provide the absolute path of the directory:
Python Program
import os
os.mkdir('D:\workspace\sample')
print('The directory is created.')
Explanation (Step-by-Step):
- Import the
os
module. - Use
os.mkdir()
with the absolute path'D:\workspace\sample'
to create the directory in the specified location. - If the operation is successful, the directory will be created at the given path.
- Print the message
'The directory is created.'
to confirm the action.
2. Create a Directory That Already Exists
If you attempt to create a directory that already exists, Python raises a FileExistsError
.
Python Program
import os
os.mkdir('sample')
print('The directory is created.')
Output
Traceback (most recent call last):
File "example.py", line 3, in
os.mkdir('sample')
FileExistsError: [WinError 183] Cannot create a file when that file already exists: 'sample'
Explanation (Step-by-Step):
- Import the
os
module. - Attempt to create a directory named
sample
usingos.mkdir()
. - Since the directory already exists, Python raises a
FileExistsError
. - The error message specifies the name of the directory that could not be created and provides the relevant error code (
WinError 183
).
To avoid this error, you can check whether the directory exists before attempting to create it:
Python Program
import os
dirPath = 'C:/workspace/python/sample'
if not os.path.isdir(dirPath):
print('The directory is not present. Creating a new one..')
os.mkdir(dirPath)
else:
print('The directory is present.')
Explanation (Step-by-Step):
- Import the
os
module. - Define the desired directory path in the variable
dirPath
. - Use
os.path.isdir()
to check whether the directory already exists: - If the directory does not exist, print
'The directory is not present. Creating a new one..'
and callos.mkdir()
to create it. - If the directory exists, print
'The directory is present.'
. - This approach prevents errors and ensures that the program handles both scenarios gracefully.
Summary
In this tutorial, we learned how to create a new directory using Python's os.mkdir()
function, including handling cases where the directory already exists.