Remove Directory in Python
Python - Remove Directory
To remove a director in Python, import shutil library, call shutil.rmtree() function, and pass the directory path as argument.
The syntax to call shutil.rmtree() function to remove a directory is
import shutil
shutil.rmtree('path_to_directory')
All the files and sub-directories would be lost.
Example
In the following program, we will remove the directory "myfolder"
Directory
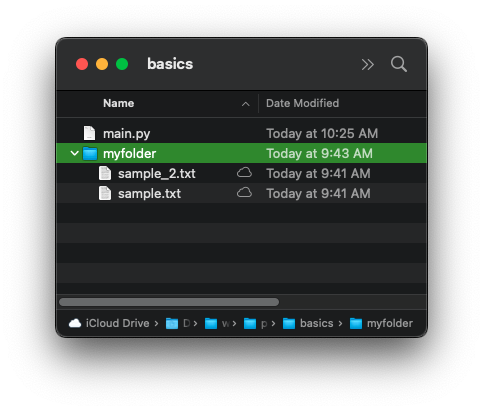
Python Program
import shutil
directory_path = 'myfolder'
try :
shutil.rmtree(source, target)
print('Directory removed successfully.')
except:
print('Could not remove directory.')
print('An exception occurred.')
Output
Directory removed successfully.
After Directory Removed
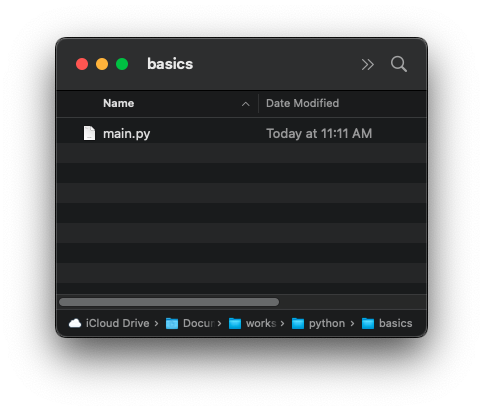
Summary
In this tutorial of Python Examples, we learned how to remove or delete a directory using shutil.rmtree() function, with the help of examples.