Read a File Line by Line in Python
To read a file line by line in Python,
- Open the file in read mode using open() built-in function.
- Use a For loop, with file object as iterator, and in each iteration, you get access to a line in the file.
The syntax to open a file in read mode is
open(file_path, "r")
The syntax to iterate over lines of a file is
for line in file:
print(line.strip())
Please note that the trailing new line character of each line is also returned. You may use String.strip() method, or some other means to remove that trailing new line character.
Examples
1. Read a given text file line by line in Python
In the following program, we shall open a file named “data.txt” in read mode.
We shall use a For loop to iterate over the file object. The iterator returns a line during each iteration in the For loop.
Python Program
file_path = "data.txt"
with open(file_path, "r") as file:
for line in file:
print(line.strip())
Output
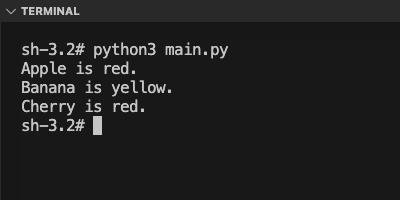
2. Print line number as well while reading the file line by line
Let us print
Python Program
file_path = "data.txt"
with open(file_path, "r") as file:
i = 0
for line in file:
i += 1
print(f"Line {i} : {line.strip()}")
Output
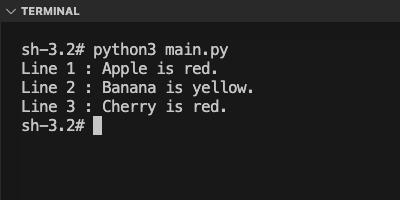
Summary
In this tutorial of Python File Operations, we have seen how to read a file line by line in Python using open() built-in function and a For loop, with examples.