Python OpenCV - Add or Blend Two Images
Python OpenCV - Add or Blend Two Images
You can add or blend two images. Blending adds the pixel values of
Using opencv, you can add or blend two images with the help of cv2.addWeighted() method.
Syntax of addWeighted()
Following is the syntax of addWeighted() function.
dst = cv.addWeighted(src1, alpha, src2, beta, gamma[, dst[, dtype]])
where src1 and src2 are input image arrays and alpha, beta are the corresponding weights to be considered while performing the weighted addition. gamma is static weight that will be added to all the pixels of the image.
addWeighted() function returns numpy array containing pixel values of the resulting image.
At a pixel level addWeighted() function performs the following operation.
dst = scr1*alpha + scr2*beta + gamma
Examples
1. Add or blend two images with OpenCV
In the following example, we will take two images, and add them together with an alpha and beta of 0.5.
Python Program
import cv2
# read two images
src1 = cv2.imread('image1.png', cv2.IMREAD_COLOR)
src2 = cv2.imread('image2.png', cv2.IMREAD_COLOR)
# add or blend the images
dst = cv2.addWeighted(src1, 0.5, src2, 0.5, 0.0)
# save the output image
cv2.imwrite('image.png', dst)
scr1 Image: image1.png
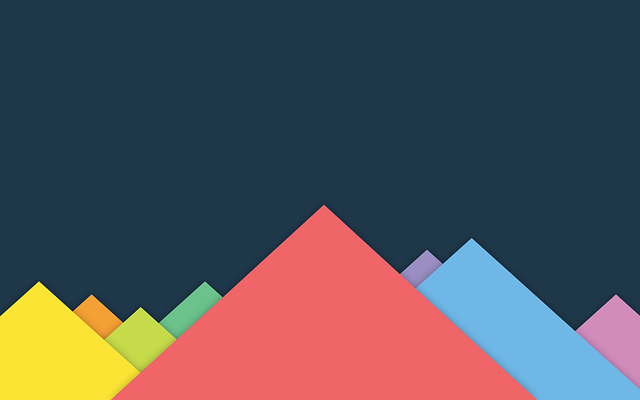
scr2 Image: image2.ong
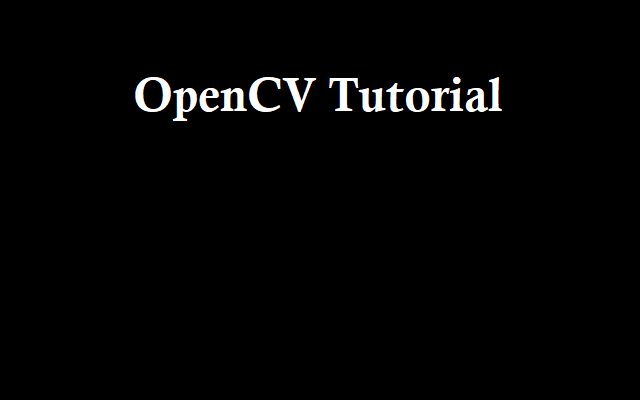
Output Image: image.png
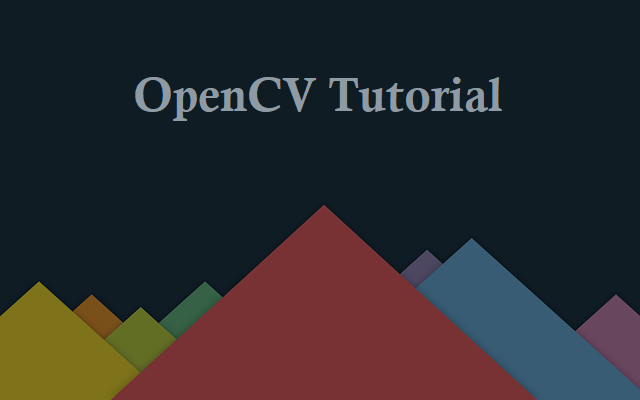
As we have provided weight of 0.5 to each of the images, the output images looks dull or darkened. In our next example, we shall try with different weights.
2. Add or blend two images with different weights
In this example, we will use the same input images as that of previous example, but different weights. alpha=1
and beta=1
.
Python Program
import cv2
# read two images
src1 = cv2.imread('image1.png', cv2.IMREAD_COLOR)
src2 = cv2.imread('image2.png', cv2.IMREAD_COLOR)
# add or blend the images
dst = cv2.addWeighted(src1, 1, src2, 1, 0.0)
# save the output image
cv2.imwrite('image.png', dst)
Output image: image.png
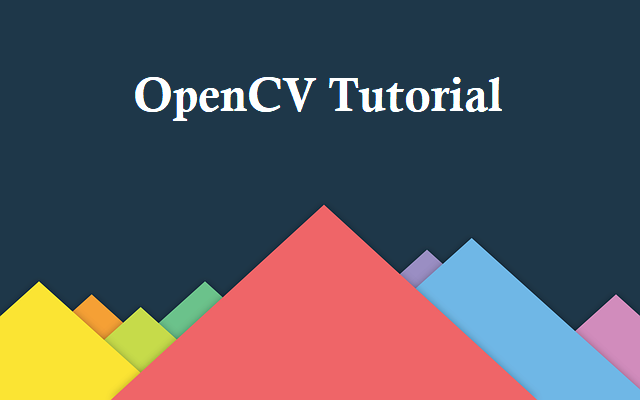
Observe the differences between output images in this and previous examples.
Summary
In this OpenCV Tutorial, we learned how to add or blend two images using OpenCV Library, with the help of example programs.