Add Noise to Image with OpenCV
Python OpenCV - Add Noise to Image
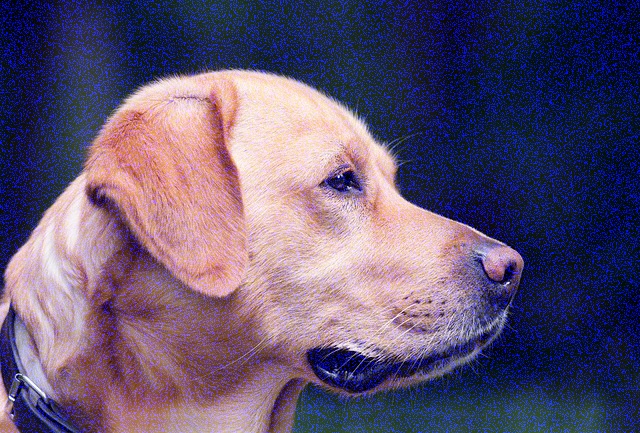
What is noise?
Noise refers to unwanted or random fluctuations in the pixel values of an image. Noise can be caused by various factors such as the imaging sensor, electrical interference, or transmission errors.
What does noise do to an image?
Noise can have a significant impact on the quality and usefulness of images. It can reduce the sharpness and contrast of an image, introduce artifacts, and make it difficult to extract meaningful information.
Adding noise is required
How to add noise to an image with OpenCV?
To add noise to an image with OpenCV in Python, you can create a gaussian noise and add this noise to the image using cv2.add()
function.
In this tutorial, you will learn how to add Gaussian noise to a given image, with examples.
Steps to add noise to an image
- Read an image into an array using cv2.imread() function.
- Generate a random Gaussian noise using
cv2.randn()
, an array whose size is same as that of given input image, but with Gaussian noise - Add this generated Gaussian noise array to the input image array using
cv2.add()
function. The function returns a noisy image. - Save the noisy image using cv2.imwrite() function.
Examples
In the following examples, we shall use the below image as input for adding noise.
test_image.jpg
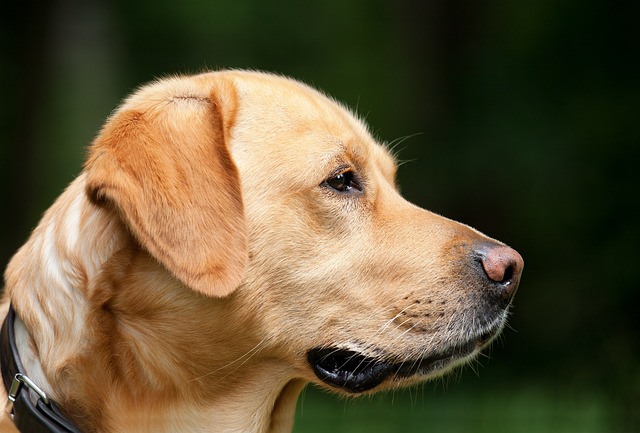
1. Add Gaussian noise to image
In the following program, we have used cv2.randn()
function to generate a Gaussian noise whose distribution is defined by a mean value, and standard deviation value. We then add this noise to the image, and save the noisy image.
Python Program
import cv2
import numpy as np
# Load the image
img = cv2.imread('test_image.jpg')
# Generate random Gaussian noise
mean = 0
stddev = 180
noise = np.zeros(img.shape, np.uint8)
cv2.randn(noise, mean, stddev)
# Add noise to image
noisy_img = cv2.add(img, noise)
# Save noisy image
cv2.imwrite('noisy_img.jpg', noisy_img)
noisy_img.jpg
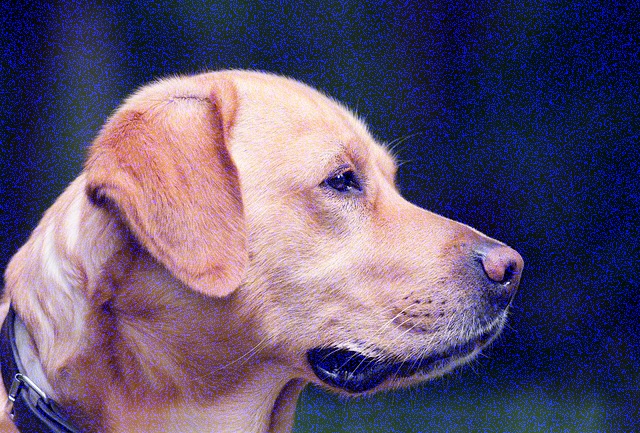
Use cases for adding noise to image
Adding noise to an image can be useful in various cases such as:
- Testing image processing algorithms: Adding noise to images can help in testing the performance of image processing algorithms such as denoising, segmentation, and feature detection under different levels of noise.
- Augmenting image datasets: Adding noise to images can help in augmenting image datasets for training machine learning models. It can improve the robustness of models and make them more resistant to noise and other variations.
- Simulating real-world conditions: In some cases, adding noise to images can help in simulating real-world conditions. For example, in medical imaging, adding noise to images can help in simulating the noise and artifacts that are often present in real medical images.
- Enhancing image aesthetics: In some cases, adding noise to images can be used to enhance their aesthetics. For example, adding film grain to digital images can help in simulating the look and feel of traditional film photography.
Summary
In this Python OpenCV Tutorial, we added noise to an image using cv2.randn()
and cv2.add()
functions, with the help of examples.