Python OpenCV CV2 - Capture Video from Camera
Python OpenCV - Capture Video from Camera
You can record a video from Camera in Python using OpenCV Library.
In this tutorial, we shall learn how to record or capture a video
Steps to Record Video from Camera
To capture video from Camera using OpenCV cv2 library, follow these steps:
- Import cv2 library.
- Get Video Capture object for a camera using cv2.VideoCapture(). You can pass the camera index if there are multiple cameras connected to the computer.
- Set up a infinite while loop.
- In the while loop, read a frame from video capture object using its read() method.
- Show the frame in a window with cv2.imshow().
- You can setup to break the loop when user clicks in a specific key.
- At the end of your video capture, release the camera and destroy all the windows generated by cv2.imshow().
Examples
1. Capture Video using Python OpenCV cv2 library from Webcam
In the following example, we have passed index as 0 to VideoCapture class. This will bring up the primary and only camera connected to this computer, i.e., webcam.
Python Program
import cv2
# capture frames from a camera with device index=0
cap = cv2.VideoCapture(0)
# loop runs if capturing has been initialized
while(1):
# reads frame from a camera
ret, frame = cap.read()
# Display the frame
cv2.imshow('Camera',frame)
# Wait for 25ms
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# release the camera from video capture
cap.release()
# De-allocate any associated memory usage
cv2.destroyAllWindows()
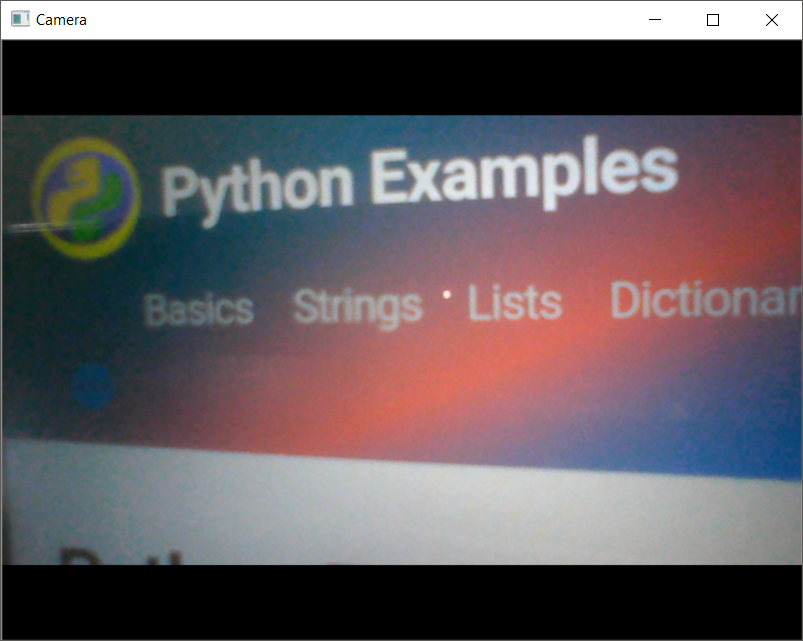
I just captured my phone from the webcam.
Summary
In this OpenCV Tutorial, we learned how to use webcam to capture an image using OpenCV, with the help of example programs.