Python Opencv - cv2.blur() - Image Blurring
Python OpenCV cv2.blur()
You can blur an image in Python using OpenCV cv2.blur() function. Also, you can blur an image using cv2.filter2D(). But, cv2.blur() is a quick high level function for filtering action and performing an averaging.
In this tutorial, we will learn how to blur an image using cv2.blur() function with examples.
Examples cover use cases where we use kernels of different shapes and its effect on the blur in the output.
Following is a quick code snippet that blurs an image img_src
with the kernel of shape (5, 5)
and returns the blurred image.
img_rst = cv2.blur(img_src, (5, 5))
In the above statement, we store the blurred image in img_rst
.
Examples
1. Blur Image - cv2.blur()
Following is a simple example, where shall blur an image and save it. The step by step process is given below.
- Read an image into
img_src
. - Blur the image with kernel of shape (5, 5).
- Store the returned image from cv2.blur() and save it to persistent storage.
Python Program
import cv2
#read image
img_src = cv2.imread('sample.png')
#blur the image
img_rst = cv2.blur(img_src, (5, 5))
#save result image
cv2.imwrite('result.jpg',img_rst)
Output
Run the above program. Following is a sample output with the source and result images specified.
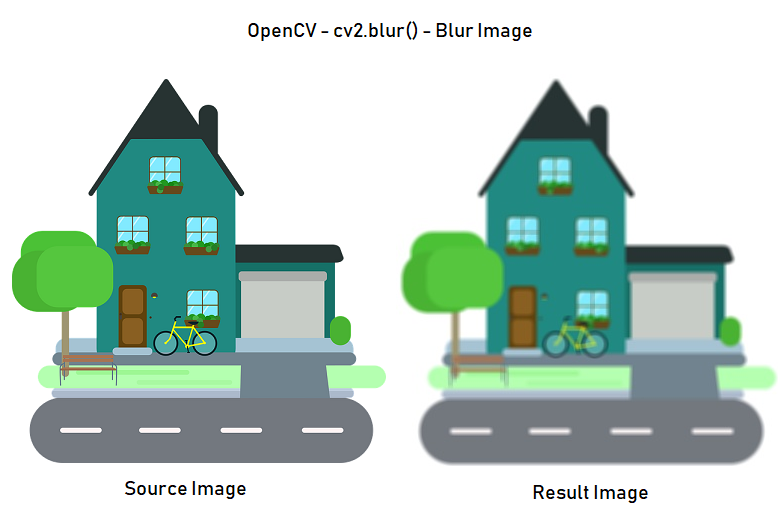
2. Blur Image with a kernel (20,20)
In our previous example, we used a kernel of shape (5, 5). In this example, we shall use a kernel of shape (20, 20) and observe the resulting image.
Python Program
import cv2
#read image
img_src = cv2.imread('sample.png')
#blur the image
img_rst = cv2.blur(img_src, (20, 20))
#save result image
cv2.imwrite('result.jpg',img_rst)
Output
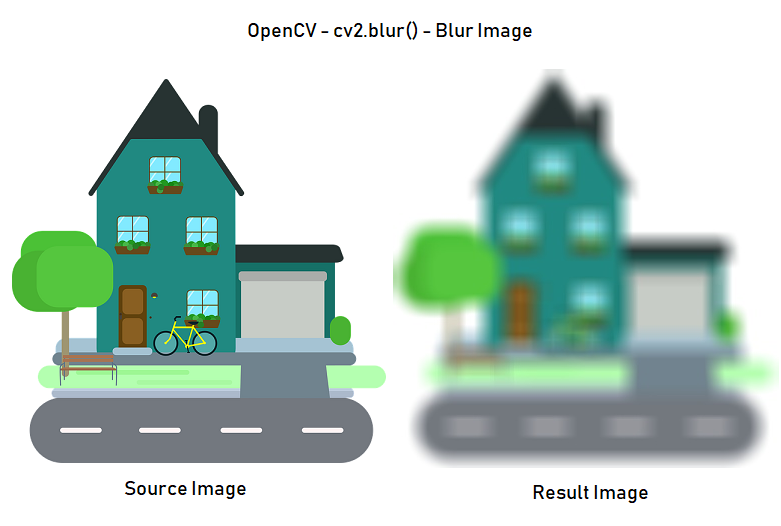
Summary
In this tutorial of Python Examples, we learned how to blur an image using cv2.blur() function of Python OpenCV cv2 library.