Python OpenCV - Corner Detection
Python OpenCV - Corner Detection
Corner detection is a fundamental technique in computer vision used to identify interesting points in an image, specifically points where there are significant changes in intensity or color.
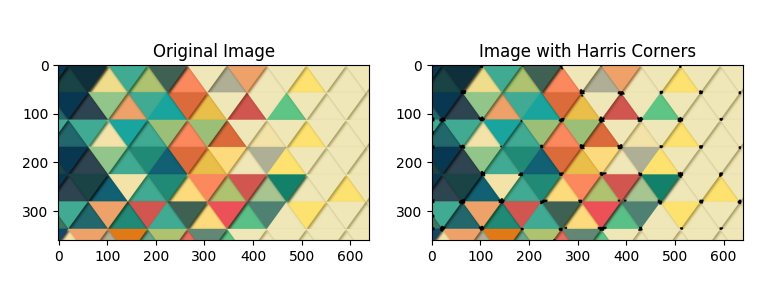
OpenCV provides several methods for corner detection, and one popular algorithm is the Harris corner detection algorithm.
In this tutorial, we shall learn how to use Harris corner detection algorithm to find corners in an image using cv2.cornerHarris()
function.
Steps for Corner Detection using OpenCV
- Given an input image.
- Read input image.
- Convert the given image to grayscale image.
- Convert this grayscale image to float32. The Harris corner detection algorithm involves computing derivatives and performing matrix operations on the image. Using floating-point precision (specifically,
float32
in this case) helps prevent issues related to overflow and loss of precision during these computations. - Call
cv2.cornerHarris()
function to compute the Harris corner response. The syntax and description of the parameters is given below. - The response is dilated to mark the corners and enhance their visibility.
- A threshold is applied to identify significant corners.
- The corners are marked in black on a copy of the original image for visualization.
- The original and marked images are displayed using Matplotlib.
Example for Corner Detection using Harris corner detection algorithm
Python Program
import cv2
import numpy as np
import matplotlib.pyplot as plt
image_file_path = 'input_image.png'
# Read the image
img = cv2.imread(image_file_path)
# Convert to grayscale
img_grayscale = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
# Convert the image to float32
img_float32 = np.float32(img_grayscale)
# Apply the Harris corner detection
dst = cv2.cornerHarris(img_float32, blockSize=5, ksize=3, k=0.04)
# Dilate to mark the corners and enhance visualization
dst = cv2.dilate(dst, None)
# Threshold for an optimal value (adjust as needed)
threshold_value = 0.01 * dst.max()
# Ensure that img_with_corners is a copy of the original image with three channels
img_with_corners = img.copy()
# Mark the corners on the image
img_with_corners[dst > threshold_value] = [0, 0, 0] # Mark corners
# Display the original and marked images
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(cv2.cvtColor(img_with_corners, cv2.COLOR_BGR2RGB))
plt.title('Image with Harris Corners')
plt.show()
Result
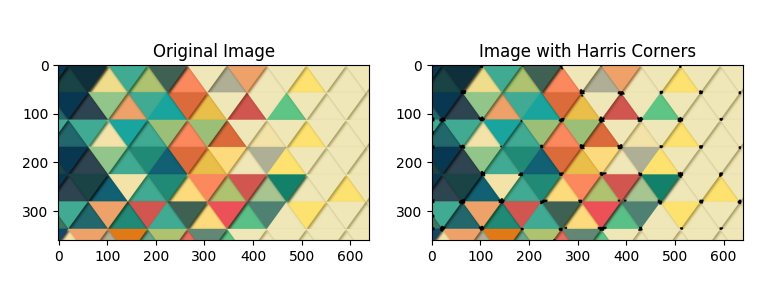
About cv2.cornerHarris() function
The syntax of cornerHarris() function is
cv2.cornerHarris(src, blockSize, ksize, k)
where
- src: This is the input single-channel grayscale image (float32 type) on which corner detection will be performed.
- blockSize: It is the size of the neighborhood considered for corner detection. It is a parameter that represents the size of the neighborhood for the covariance matrix calculation. Common values are 3, 5, or 7.
- ksize: Aperture parameter for the Sobel() operator. It represents the size of the Sobel kernel used for computing image gradients. It is the size of the kernel used for derivative calculations. Common values are 3 or 5.
- k: Harris detector free parameter in the equation. It is a constant multiplier for the computed corner response. Values between 0.04 and 0.06 are often used.