OpenCV imshow() – Display or Show Image
You can display an image to the user during the execution of your Python OpenCV application.
To display an image using opencv cv2 library, you can use cv2.imshow() function.
The syntax of imshow() function is given below.
cv2.imshow(window_name, image)
where window_name
is the title of the window in which the image
numpy.ndarray will be shown. If a window is not created already, a new window will be created to fit the image.
In this tutorial, we will go through different scenarios where we shall display an image in a window.
Examples
1. Show image using cv2.imshow()
In this example, we read an image from storage and display the image to the user in a window.
Python Program
import cv2
#read image
img = cv2.imread('D:/my-image.png')
#show image
cv2.imshow('Example - Show image in window',img)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
cv2.waitKey(0) is important for holding the execution of the python program at this statement, so that the image window stays visible. If you do not provide this statement, cv2.imshow() executes in fraction of a second and the program closes all the windows it opened, which makes it almost impossible to see the image on the window.
Output
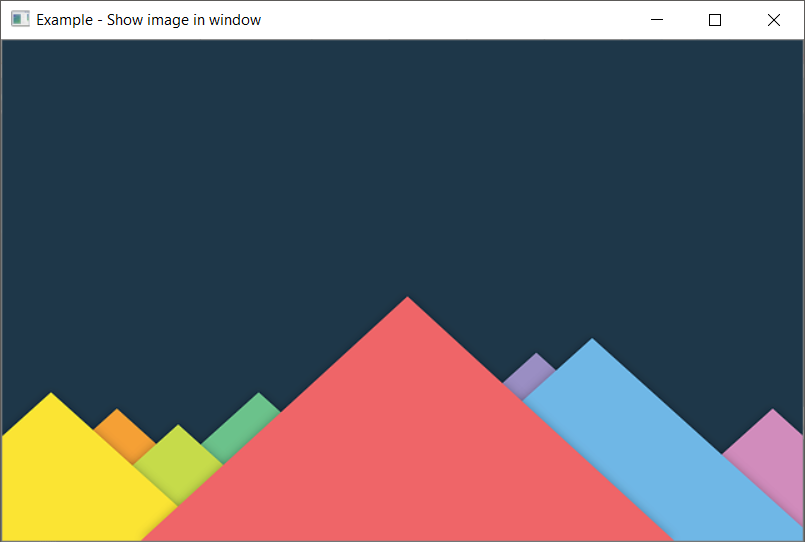
A window is opened to show the image. Also, the window size is such that it fits the image. If the image size is greater than your screen resolution, then a window is opened with the scaled down version of the image.
2. Show numpy.ndarray as image using OpenCV
In this example, we try to show an ndarray as image using imshow(). We initialize a numpy array of shape (300, 300, 3) such that it represents 300×300 image with three color channels. 125 is the initial value, so that we get a mid grey color.
Python Program
import cv2
import numpy as np
#numpy array
ndarray = np.full((300,300,3), 125, dtype=np.uint8)
#show image
cv2.imshow('Example - Show image in window', ndarray)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
Output
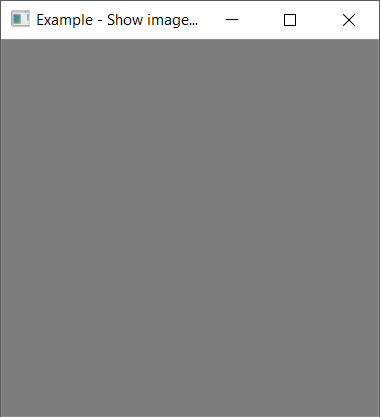
Summary
In this tutorial of Python Examples, we learned how to show or display an image in a window to user using cv2.imshow().