OpenCV cv2.imwrite() - Save Image - Python Examples
Python OpenCV cv2.imwrite()
In our previous tutorial - cv2 imread(), we learned to read an image into a matrix. You may transform this matrix by using some algorithms. Then it may be required to save this matrix as an image.
In this tutorial, we will learn how to save an array as image in file system.
To save image to local storage using Python, use cv2.imwrite() function on OpenCV library.
Syntax of cv2 imwrite()
The syntax of imwrite() function is:
cv2.imwrite(path, image)
where path is the complete path of the output file to which you would like to write the image numpy array.
cv2.imwrite() returns a boolean value. True if the image is successfully written and False if the image is not written successfully to the local path specified.
Examples
1. Save matrix as image using cv2.imwrite()
In this example, we will read an image, transform it and then save the image to persistent file storage using imwrite() method.
Python Program
import cv2
#read image as grey scale
img = cv2.imread('D:/image-1.png')
#do some transformations on img
#save matrix/array as image file
isWritten = cv2.imwrite('D:/image-2.png', img)
if isWritten:
print('Image is successfully saved as file.')
Explanation
- The program imports the required
cv2
module for image processing tasks. cv2.imread('D:/image-1.png')
reads the image from the specified file path into a matrix/array. Since no flag is specified, the image is read in color mode by default.- Image transformations can be applied to the
img
variable, but this step is only commented and not executed in the code. cv2.imwrite('D:/image-2.png', img)
writes the matrix/arrayimg
to the specified file path as a PNG image.- The function returns
True
if the image is successfully saved, and the result is stored inisWritten
. - If
isWritten
isTrue
, the program prints'Image is successfully saved as file.'
to confirm that the operation succeeded.
Output
Image is successfully saved as file.
2. Save image created with random pixel values using cv2.imwrite()
In this example, we will write a numpy array as image using cv2.imwrite() function. For that, we will create a numpy array with three channels for Red, Green and Blue containing random values.
In general cases, we read image using cv2.imread(), apply some transformations on the array and then write the image to the local storage. But in this example, we will stick to the array with random values.
Python Program
import cv2
import numpy as np
img = np.random.randint(255, size=(300, 600, 3))
isWritten = cv2.imwrite('D:/image-2.png', img)
if isWritten:
print('The image is successfully saved.')
Explanation
- The program imports the required modules
cv2
andnumpy
. np.random.randint(255, size=(300, 600, 3))
creates a random 3-channel image of dimensions 300x600, where pixel values range from 0 to 254.cv2.imwrite('D:/image-2.png', img)
writes the generated image to the specified file path as a PNG file.- The result of the
imwrite
function is stored in the variableisWritten
, which will beTrue
if the image is saved successfully. - If
isWritten
isTrue
, the program prints the message'The image is successfully saved.'
to confirm the operation.
Output
The image is successfully saved.
Following is the image that is generated with the random values.
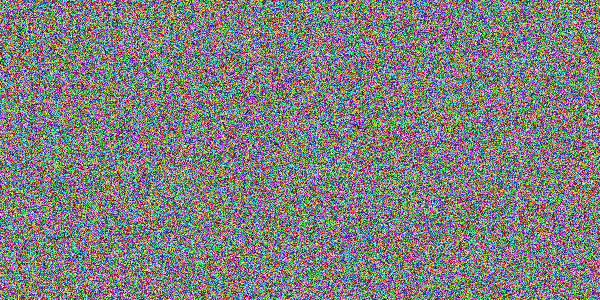
Summary
In this Python OpenCV Tutorial, we have seen how to use cv2.imwrite() to save numpy array as an image.