OpenCV – Get Blue Channel from Image
To extract blue channel of image, first read the color image using Python OpenCV library and then extract the blue channel 2D array from the image array using image slicing.
Step by step process to extract Blue Channel of Color Image
Following is sequence of steps to get the blue channel of colored image.
- Read image using cv2.imread().
- imread() returns BGR (Blue-Green-Red) array. It is three dimensional array i.e., 2D pixel arrays for three color channels.
- Extract the blue channel alone by accessing the array.
Examples
1. Get blue color channel from image
In the following example, we shall implement the sequence of steps mentioned above, to extract the Blue Channel from the following image.
Source Image or Input Image
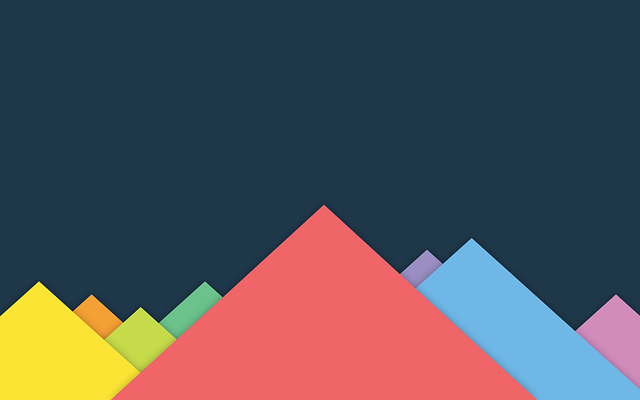
Python Program
import cv2
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
#extract blue channel
blue_channel = src[:,:,0]
#write blue channel to greyscale image
cv2.imwrite('D:/cv2-blue-channel.png',blue_channel)
We have written the blue channel to an image. As this is just a 2D array with values ranging from 0 to 255, the output looks like a greyscale image, but these are blue channel values.
Output Image (Blue Channel)
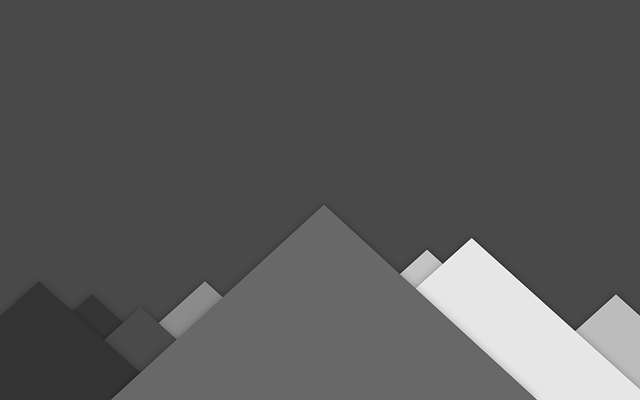
If you observe the triangles, the red and green ones are more darker than the other.
To visualize the image in blue color, let us make the red and green components to zeroes.
Python Program
import cv2
import numpy as np
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
# extract blue channel
blue_channel = src[:,:,0]
# create empty image with same shape as that of src image
blue_img = np.zeros(src.shape)
#assign the red channel of src to empty image
blue_img[:,:,0] = blue_channel
#save image
cv2.imwrite('D:/cv2-blue-channel.png',blue_img)
Output Image
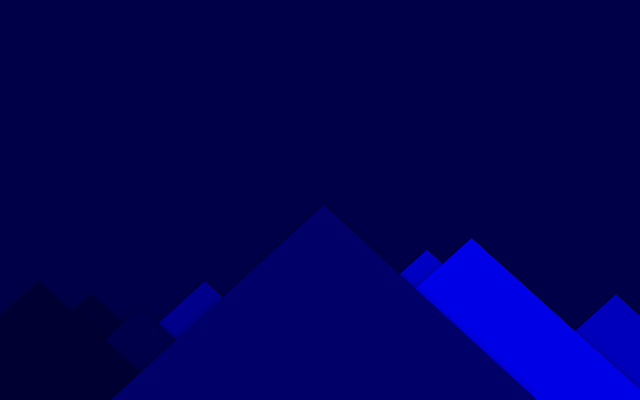
Summary
In this Python OpenCV Tutorial, we learned how to extract blue channel from an image.