Python OpenCV – Histogram Equalization
Histogram equalization is a technique used to enhance the contrast of an image by adjusting the distribution of pixel intensities.
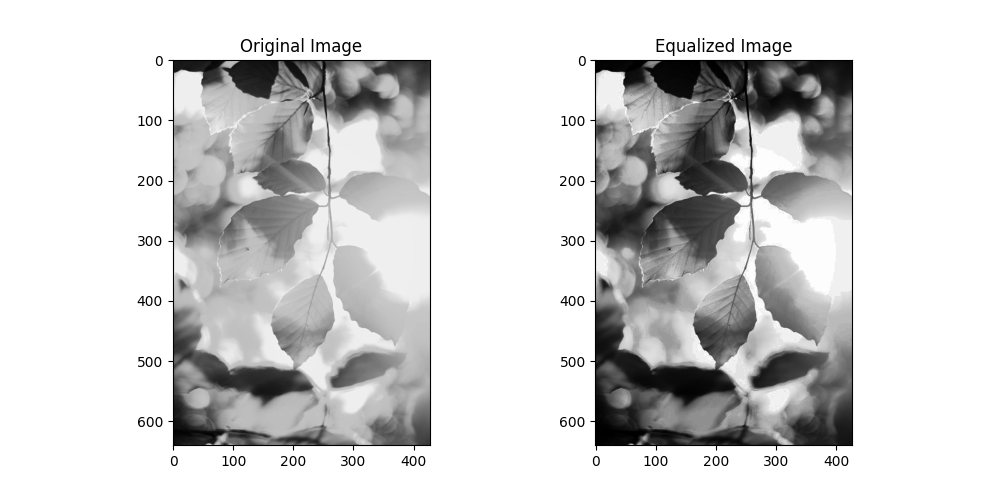
In OpenCV, you can perform histogram equalization using the cv2.equalizeHist()
function.
Keep in mind that histogram equalization is often more effective on grayscale images. If you have a color image, you may want to convert it to grayscale before applying histogram equalization.
Example for Histogram Equalization using OpenCV
In this example:
- Given an input color image.
- Read the image image as a grayscale image using cv2.imread() function.
- Apply
cv2.equalizeHist()
function to the grayscale version of the image. - Display the original and equalized images side by side using Matplotlib.
If you do not have matplotlib installed, run the command pip install matplotlib
or pip3 install matplotlib
in the terminal or command prompt to install the matplotlib library.
Python Program
import cv2
import matplotlib.pyplot as plt
image_file_path = 'input_color_image.jpg'
# Convert to grayscale if input is color
grayscale_img = cv2.imread(image_file_path, cv2.IMREAD_GRAYSCALE)
# Apply histogram equalization
equalized_img = cv2.equalizeHist(grayscale_img)
#cv2.imwrite('output_image.jpg', equalized_img)
# Display the original and equalized images
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.imshow(grayscale_img, cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(equalized_img, cmap='gray')
plt.title('Equalized Image')
plt.show()
Result
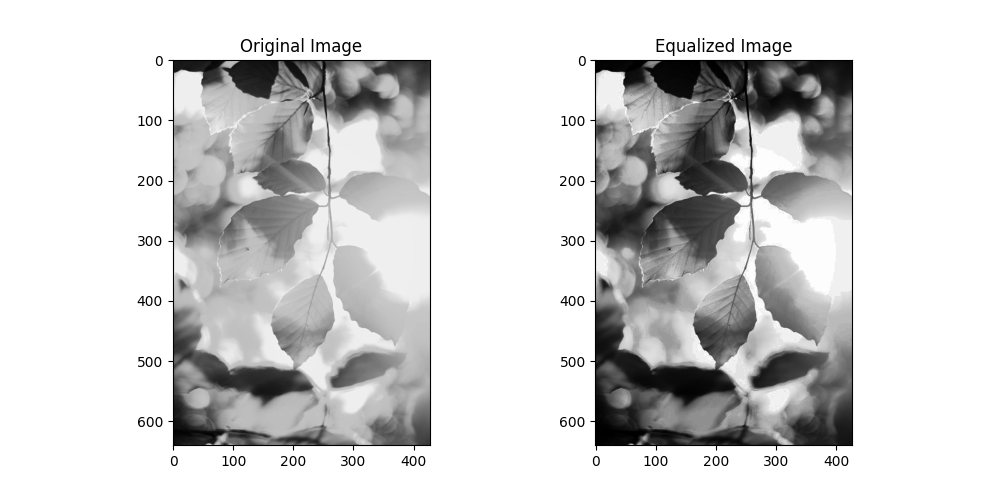
Note: Histogram equalization may not always produce desirable results, especially in cases where the image already has a well-distributed histogram. It’s essential to evaluate the impact of histogram equalization on different types of images.