Python OpenCV – Convert JPG to WebP
To convert an image from JPG to WebP using OpenCV in Python, you can use the cv2
library imread() and imwrite() functions.
Steps to convert JPG to WebP using OpenCV
- Given JPG image file path in
jpg_file_path
. - Read image using cv2.imread() function. The imread() function returns a MatLike image object.
- Take a WebP file path in
webp_file_path
. We shall save the image object to this file path. - Call cv2.imwrite() function, and pass the WebP file path and the input image object as first two arguments respectively. Also, for the
params
, the third parameter, we shall specify the quality with which the WebP image has to be saved using cv2.IMWRITE_WEBP_QUALITY.
Examples
Example 1: Convert JPG to WebP with specific quality
In this example, we shall take the following JPG image, and convert this image to WebP with 80% quality. You may change the quality based on your requirement. If you decrease the quality, the file size of the resulting WebP also usually becomes less. The quality can be given in the range [0, 100].
input_image.jpg
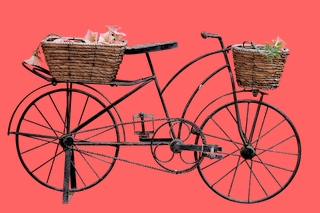
Python Program
import cv2
jpg_file_path = 'input_image.jpg'
webp_file_path = 'output_image.webp'
# Read the JPG image
jpg_img = cv2.imread(jpg_file_path)
# Save the image in JPG format
cv2.imwrite(webp_file_path, jpg_img, [int(cv2.IMWRITE_WEBP_QUALITY), 80])
Output image
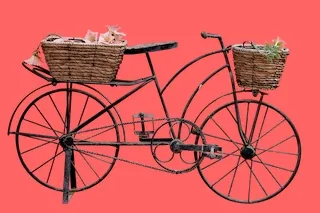
In this example, the file size of input JPG image is 58KB, while that of the output WebP image is 13KB.
Online Image Conversion Tools
If you would like to convert an image from JPG to WebP online, refer the following page.
Convert JPG to WebP by ConvertOnline.org.
For conversion between other formats online, refer Online Image Converter.
Summary
In this tutorial, we have learnt how to convert a given JPG image to WEbP image using OpenCV cv2 library.