Python OpenCV - Write Text on Image - putText()
Write Text on Image in Python
To write text on image with OpenCV library of Python, use putText() method.
Examples
1. Write text on image using putText()
In the following program, we write text on the image from the position (10, 50) i.e., 10 pixels from the top, and 50 pixels from the left.
Python Program
import numpy as np
import cv2
image = cv2.imread('sample.png',cv2.IMREAD_UNCHANGED)
position = (10,50)
cv2.putText(
image, #numpy array on which text is written
"Python Examples", #text
position, #position at which writing has to start
cv2.FONT_HERSHEY_SIMPLEX, #font family
1, #font size
(209, 80, 0, 255), #font color
3) #font stroke
cv2.imwrite('output.png', image)
As you can see from the above example, you can provide
- image on which you can write the text.
- text you want to write on image.
- position: distance along horizontal and vertical axis from top left corner of the image.
- font family
- font size
- font color
- font stroke width
In the above example, we have provided a sample image, and wrote some text on the image. We have written the output image to a file. Following are the input and output images.
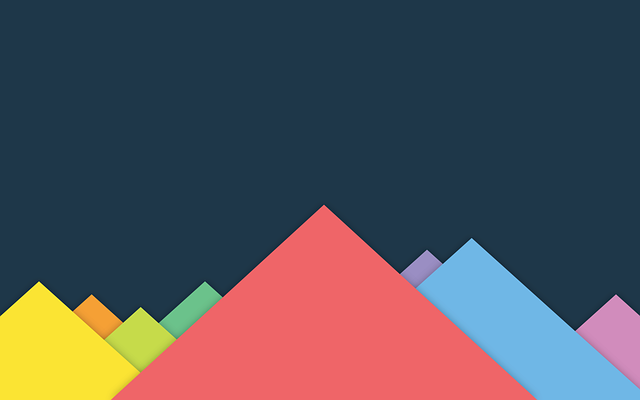
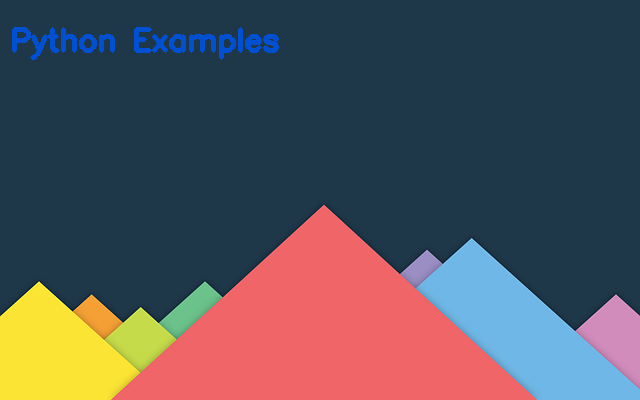
2. Write text at the center of the image
If you know the shape (width, height) of the text you are writing on the image, then you can place at center aligned on the image.
The approximate shape of the text in the above example is (268, 36)
. You may have to find the shape of your specific text this using Paint or other application.
Python Program
import numpy as np
import cv2
image = cv2.imread('sample.png',cv2.IMREAD_UNCHANGED)
position = ((int) (image.shape[1]/2 - 268/2), (int) (image.shape[0]/2 - 36/2))
cv2.putText(
image,
"Python Examples",
position,
cv2.FONT_HERSHEY_SIMPLEX,
1, #font size
(209, 80, 0, 255), #font color
3) #font stroke
cv2.imwrite('output.png', image)
The output image would be:
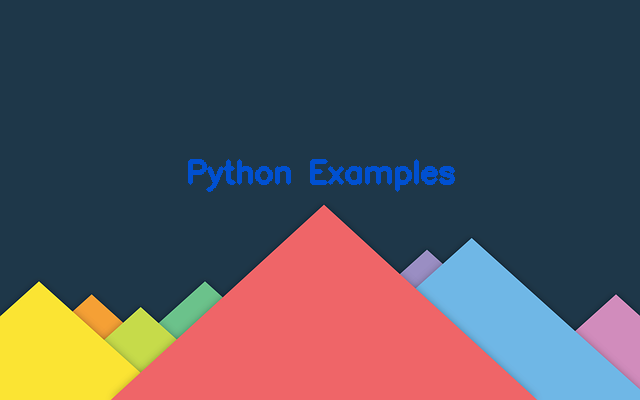
Summary
In this Python OpenCV tutorial, we learned how to write text on an image using cv2.put_text().