OpenCV – Convert Colored Image to Binary Image
At times, you may need to convert an image to a binary image. In other words, you need to convert a color image or greyscale image to black and white image.
In this tutorial, we shall learn how to convert an image from color to black and white.
Steps to convert coloured image to binary image
Converting an image to black and white involves two steps.
- Read the source image as grey scale image.
- Convert the grey scale image to binary with a threshold of your choice.
If your source image is grey scale image, then you can read the image in step#1 as original image and continue with step#2. Following example illustrates the working of threshold when converting from grey scale to binary or black and white.
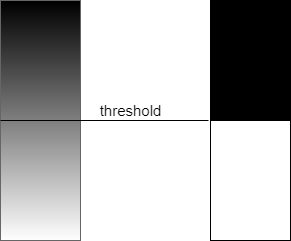
Examples
1. Convert color image to black and white
In the following example, we will read the following color image using cv2.imread() as grey scale image and then apply cv2.threshold() function on the image array.
Input Image
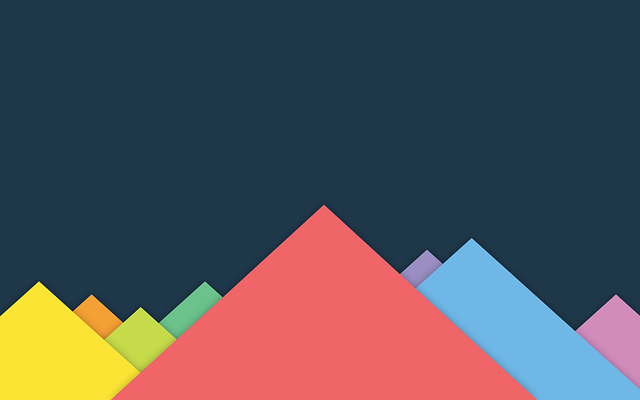
Python Program
import cv2
#read image
img_grey = cv2.imread('D:/original.png', cv2.IMREAD_GRAYSCALE)
# define a threshold, 128 is the middle of black and white in grey scale
thresh = 128
# threshold the image
img_binary = cv2.threshold(img_grey, thresh, 255, cv2.THRESH_BINARY)[1]
#save image
cv2.imwrite('D:/black-and-white.png',img_binary)
The output black-and-white.png is as shown below.
Output Binary Image
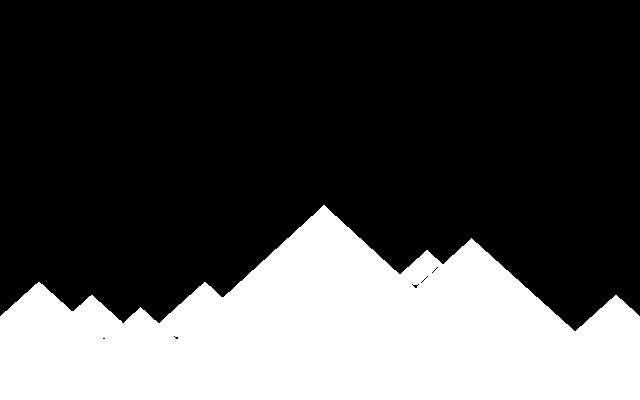
2. Convert grey scale image to black and white
In the following example, we will read a grey scale image using cv2.imread() and then apply cv2.threshold() function on the image array. There is no difference in converting a color image to black and white and grey scale image to black and white.
Python Program
import cv2
#read image as grey scale
img_grey = cv2.imread('D:/greyscale.png', cv2.IMREAD_GRAYSCALE)
# define a threshold, 128 is the middle of black and white in grey scale
thresh = 128
# threshold the image
img_binary = cv2.threshold(img_grey, thresh, 255, cv2.THRESH_BINARY)[1]
#save image
cv2.imwrite('D:/black-and-white.png',img_binary)
Summary
In this Python OpenCV Tutorial, we learned how to convert a color image to binary or in other words black and white.