Python OpenCV – Convert PNG to JPG
To convert an image from PNG to JPG using OpenCV in Python, you can use the cv2
library imread() and imwrite() functions.
Steps to convert PNG to JPG using OpenCV
- Given PNG image file path in
png_file_path
. - Read image using cv2.imread() function. The imread() function returns a MatLike image object.
- Take a JPG file path in
jpg_file_path
. We shall save the image object to this file path as JPG. - Call cv2.imwrite() function, and pass the JPG file path and the input image object. Also, for the params, we shall specify the quality with which the JPG has to be saved: IMWRITE_JPEG_QUALITY.
Examples
Example 1: Convert PNG to JPG with specific quality
In this example, we shall take the following PNG image, and convert this image to JPG with 100% quality. You may change the quality based on your requirement. If you decrease the quality, the file size of the resulting JPG is also less. The quality can be given in the range [0, 100].
input_image.png
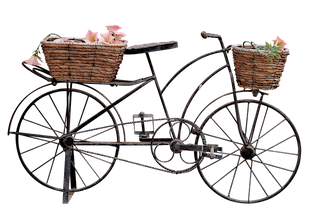
Python Program
import cv2
png_file_path = 'input_image.png'
jpg_file_path = 'output_image.jpg'
# Read the PNG image
png_img = cv2.imread(png_file_path, cv2.IMREAD_UNCHANGED)
# Save the image in JPG format
cv2.imwrite(jpg_file_path, png_img, [int(cv2.IMWRITE_JPEG_QUALITY), 100])
Output image
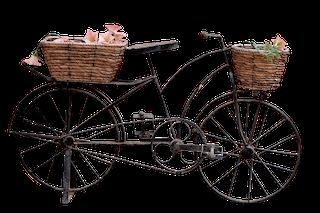
The transparency channel of the PNG has been filled with black color, and this is the default behavior.
Example 2: Convert PNG to JPG with specific background color
If you would like to specify a background color for the transparent area, create a new image with the required background color, and then composite the given PNG image on to the new image.
We shall consider the same input image as in the previous example.
Python Program
import cv2
import numpy as np
png_file_path = 'input_image.png'
jpg_file_path = 'output_image.jpg'
background_color = (100, 100, 255)
# Read the PNG image
img = cv2.imread(png_file_path, cv2.IMREAD_UNCHANGED)
# Create a new image with the desired background color
new_img = np.full_like(img, background_color + (255,), dtype=np.uint8)
# Extract the alpha channel from the original image
alpha_channel = img[:, :, 3]
# Composite the original image onto the new image using the alpha channel
for c in range(0, 3):
new_img[:, :, c] = new_img[:, :, c] * (1 - alpha_channel / 255) + img[:, :, c] * (alpha_channel / 255)
# Save the image in JPG format
cv2.imwrite(jpg_file_path, new_img, [int(cv2.IMWRITE_JPEG_QUALITY), 100])
Output image
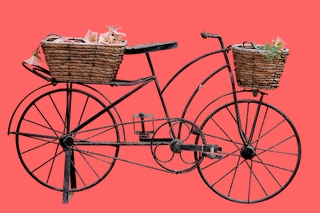
Online Image Conversion Tools
If you would like to convert an image from PNG to JPG online, refer the following page.
Convert PNG to JPG by ConvertOnline.org.
For conversion between other formats online, refer Online Image Converter.
Summary
In this tutorial, we have learnt how to convert a given PNG image to JPG image using OpenCV cv2 library.