Python OpenCV – Convert BGR to RGB color
OpenCV uses the BGR (Blue, Green, Red) color order by default. If you need to convert an image from BGR to RGB, you can use the cv2.cvtColor()
function.
Steps to convert BGR color image to RGB color image using OpenCV
- Given image file path in
image_file_path
. - Read image using cv2.imread() function. The imread() function returns a MatLike image object.
- Call
cv2.cvtColor()
function, and pass the image object and cv2.COLORBGR2RGB value. ThecvtColor()
function returns the image object in RGB color. Save it inimg_rgb
. - Now,
img_rgb
will contain the image data in RGB color order. Keep in mind that the pixel values themselves are not changed, only the order in which the color channels are arranged.
Example
Example 1: Convert image object from BGR color to RGB color
In this example, we shall take the following JPG image, read the image using imread(), and then convert the returned image object from BGR color to RGB color.
input_image.jpg
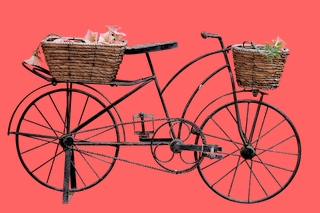
Python Program
import cv2
image_file_path = 'input_image.jpg'
# Read the image
img_bgr = cv2.imread(image_file_path, cv2.IMREAD_UNCHANGED)
# Convert BGR image to RGB image object
img_rgb = cv2.cvtColor(img_bgr, cv2.COLOR_BGR2RGB)
# Apply required transforamations on RGB image
Summary
In this tutorial, we have learnt how to convert a given image object from BGR color to an image object with RGB color using OpenCV cv2 library.