Python OpenCV – Convert PNG to WebP
To convert an image from PNG to WebP using OpenCV in Python, you can use the cv2
library imread() and imwrite() functions.
Steps to convert PNG to WebP using OpenCV
- Given PNG image file path in
png_file_path
. - Read image using cv2.imread() function. The imread() function returns a MatLike image object.
- Take a WebP file path in
webp_file_path
. We shall save the image object to this file path. - Call cv2.imwrite() function, and pass the WebP file path and the input image object. Also, for the params, we shall specify the quality with which the WebP image has to be saved: IMWRITE_WEBP_QUALITY.
Examples
Example 1: Convert PNG to WebP with specific quality
In this example, we shall take the following PNG image, and convert this image to WebP with 80% quality. You may change the quality based on your requirement. If you decrease the quality, the file size of the resulting WebP also usually becomes less. The quality can be given in the range [0, 100].
input_image.png
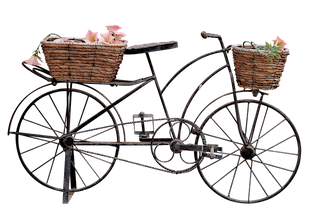
Python Program
import cv2
# Example usage:
png_file_path = 'input_image.png'
webp_file_path = 'output_image.webp'
# Read the PNG image
png_img = cv2.imread(png_file_path, cv2.IMREAD_UNCHANGED)
# Save the image in JPG format
cv2.imwrite(webp_file_path, png_img, [int(cv2.IMWRITE_WEBP_QUALITY), 80])
Output image
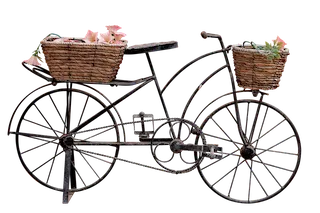
The transparency is preserved in WebP image.
In this example, the file size of input PNG image is 94KB, while that of the output WebP image is 35KB.
Online Image Conversion Tools
If you would like to convert an image from PNG to WebP online, refer the following page.
Convert PNG to WebP by ConvertOnline.org.
For conversion between other formats online, refer Online Image Converter.
Summary
In this tutorial, we have learnt how to convert a given PNG image to WEbP image using OpenCV cv2 library.