OpenCV – Extract Red Channel from Image
To extract red channel of image, we will first read the color image using cv2 and then extract the red channel 2D array from the image array.
In this tutorial, we shall learn how to extract the red channel from the colored image, by applying array slicing on the numpy array representation of the image.
Step by step process to extract Red Channel of Color Image
Following is the sequence of steps to extract red channel from an image.
- Read image using cv2.imread().
- imread() returns BGR (Blue-Green-Red) array. It is three dimensional array i.e., 2D pixel arrays for three color channels.
- Extract the red channel alone by slicing the array.
Examples
1. Get red color channel from given image
In the following example, we will implement all the steps mentioned above to extract the Red Channel from the following image.
Input Image
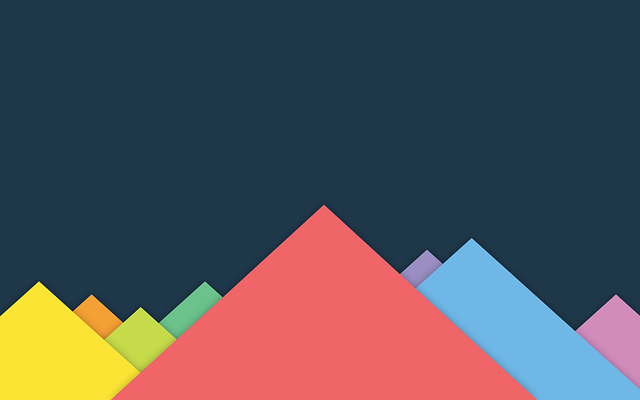
Python Program
import cv2
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
#extract red channel
red_channel = src[:,:,2]
#write red channel to greyscale image
cv2.imwrite('D:/cv2-red-channel.png',red_channel)
We have written the red channel to an image. As this is just a 2D array with values ranging from 0 to 255, the output looks like a greyscale image, but these are red channel values.
Output Image
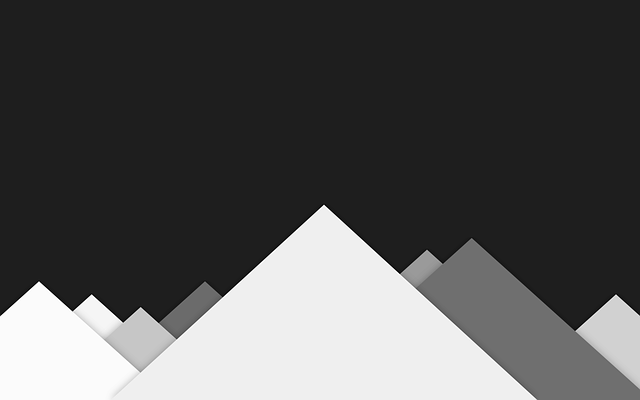
If you observe the triangles, the greener and bluer ones are more darker than the other.
To visualize the image in red color, let us make the blue and green components to zeroes.
Python Program
import cv2
import numpy as np
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
# extract red channel
red_channel = src[:,:,2]
# create empty image with same shape as that of src image
red_img = np.zeros(src.shape)
#assign the red channel of src to empty image
red_img[:,:,2] = red_channel
#save image
cv2.imwrite('D:/cv2-red-channel.png',red_img)
Output Image
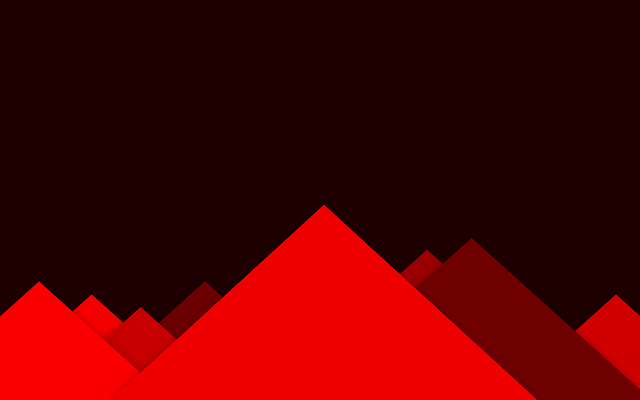
Summary
In this Python OpenCV Tutorial, we learned how to extract red channel of a colored image.