Python Remove Blue Channel from Color Image
OpenCV - Remove Blue Channel from Image
To remove blue channel from color image, read image to BGR array using cv2.imread() and assign zeros to the 2D array corresponding to blue channel.
In this tutorial, we shall use OpenCV Python library and transform an image, such that no red channel is present in the image.
Examples
1. Remove blue color channel from given image
In this example, we will remove the blue channel from the following image.
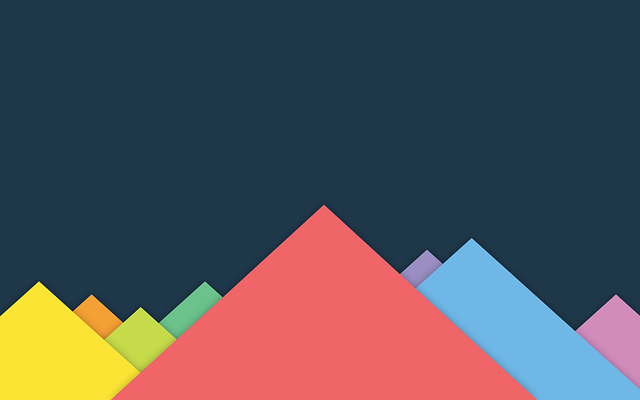
Python Program
import cv2
import numpy as np
#read image
src = cv2.imread('D:/original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
# assign blue channel to zeros
src[:,:,0] = np.zeros([src.shape[0], src.shape[1]])
#save image
cv2.imwrite('D:/no-blue-channel.png',src)
Output Image
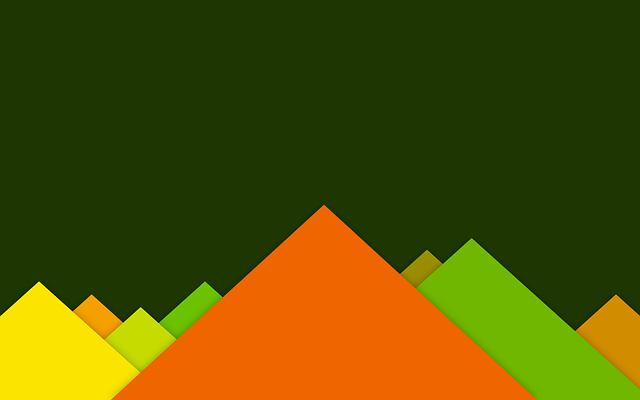
This image has only red and green channels of the source image.
Summary
In this Python OpenCV Tutorial, we learned how to remove blue channel from an image.