Convert PNG to JPG with specific background color using OpenCV in Python
To convert PNG image to JPG image with specific background color using OpenCV in Python,
- Read the given PNG image using cv2.imread() function.
- Create a new image using
np.full_like()
function, with the same dimensions as that of the given image. This image (numpy array) is filled with the specified background color. - Extract the alpha channel from the input PNG image using array slicing.
- Composite the original image onto the new image using the alpha channel.
- Write the composite image to the given JPG file path, with a specific JPG quality. You can choose the quality in the range [0, 100], where the file size is in proportion to the chosen quality.
Example
In this example, we shall consider the following image as input PNG image, and convert this PNG image to JPG image with a background color of (100, 100, 255).
input_image.png
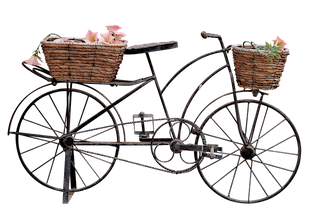
Python Program
import cv2
import numpy as np
# Example usage:
png_file_path = 'input_image.png'
jpg_file_path = 'output_image.jpg'
background_color = (100, 100, 255)
# Read the PNG image
img = cv2.imread(png_file_path, cv2.IMREAD_UNCHANGED)
# Create a new image with the desired background color
new_img = np.full_like(img, background_color + (255,), dtype=np.uint8)
# Extract the alpha channel from the original image
alpha_channel = img[:, :, 3]
# Composite the original image onto the new image using the alpha channel
for c in range(0, 3):
new_img[:, :, c] = new_img[:, :, c] * (1 - alpha_channel / 255) + img[:, :, c] * (alpha_channel / 255)
# Save the image in JPG format
cv2.imwrite(jpg_file_path, new_img, [int(cv2.IMWRITE_JPEG_QUALITY), 100])
Output image
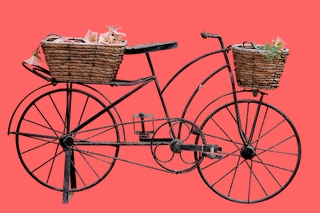