Extract Green Channel from Color Image using Python OpenCV
OpenCV - Get Green Channel from Image
To extract green channel of image, first read the color image using Python OpenCV library and then extract the green channel 2D array from the image array using image slicing.
In this tutorial, we shall learn how to extract the green channel, with the help of example programs.
Step by step process to extract Green Channel of Color Image
Following is a step by step process to extract green channel from image.
- Read image using cv2.imread().
- imread() returns BGR (Blue-Green-Red) array. It is three dimensional array i.e., 2D pixel arrays for three color channels.
- Extract the green channel alone by slicing the array.
Examples
1. Get green color channel from given image
In the following example, we shall implement all the steps mentioned above to extract the Green Channel from the following image.
Source Image or Input Image
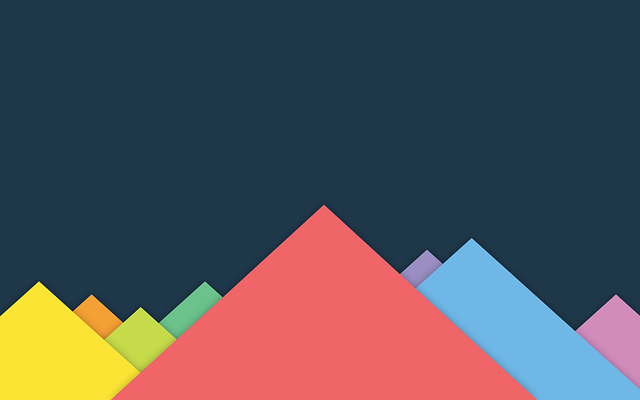
Python Program
import cv2
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
#extract green channel
green_channel = src[:,:,1]
#write green channel to greyscale image
cv2.imwrite('D:/cv2-green-channel.png',green_channel)
We have written the green channel to an image. As this is just a 2D array with values ranging from 0 to 255, the output looks like a greyscale image, but these are green channel values.
Output Image
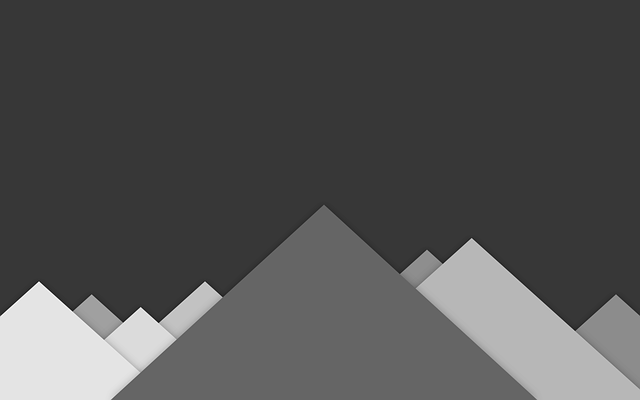
If you observe the triangles, the red and blue ones are more darker than the other.
To visualize the image in green color, let us make the red and blue components to zeroes.
Python Program
import cv2
import numpy as np
#read image
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
print(src.shape)
# extract green channel
green_channel = src[:,:,1]
# create empty image with same shape as that of src image
green_img = np.zeros(src.shape)
#assign the green channel of src to empty image
green_img[:,:,1] = green_channel
#save image
cv2.imwrite('D:/cv2-green-channel.png',green_img)
Output Image
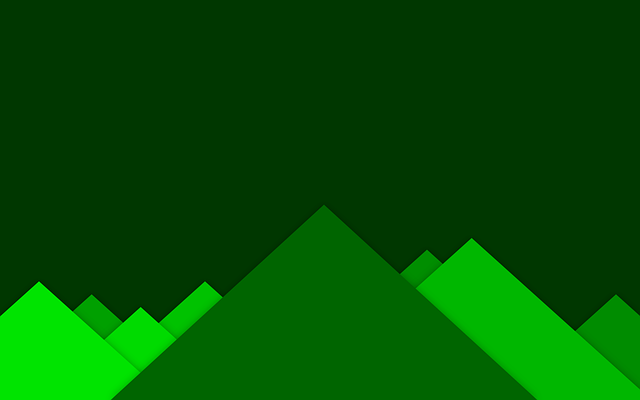
Summary
In this Python OpenCV Tutorial, we learned how to extract green channel from an image.