OpenCV cv2 Resize Image - 3 Python Examples
OpenCV cv2.resize()
To resize an image in Python, you can use cv2.resize() function of OpenCV library cv2.
Resizing, by default, does only change the width and height of the image. The aspect ratio can be preserved or not, based on the requirement. Aspect Ratio can be preserved by calculating width or height for given target height or width respectively.
In this tutorial, we shall learn how to resize image in Python using OpenCV library.
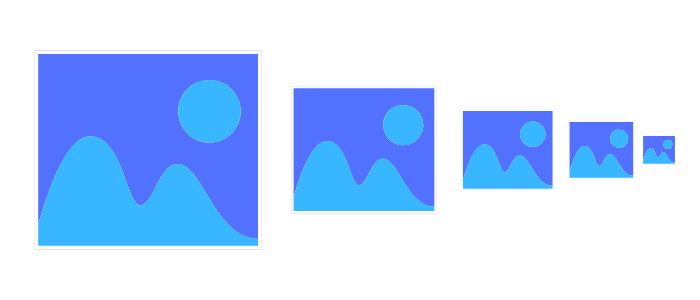
Syntax of cv2 resize() function
Following is the syntax of cv2.resize() function.
cv2.resize(src, dsize[, dst[, fx[, fy[, interpolation]]]])
where
src
is the source, original or input image in the form of numpy arraydsize
is the desired size of the output image, given as tuplefx
is the scaling factor along X-axis or Horizontal axisfy
is the scaling factor along Y-axis or Vertical axisinterpolation
could be one of the following values.- INTER_NEAREST
- INTER_LINEAR
- INTER_AREA
- INTER_CUBIC
- INTER_LANCZOS4
Based on the interpolation technique selected, respective algorithm is used. You can think interpolation as a method that decides which pixel gets which value based on its neighboring pixels and the scale at which the image is being resized.
How could you resize an image?
You can resize an image in three ways.
- Preserve the Aspect Ration and increase or decrease the width and height of the image. Just to make things clear, Aspect Ratio is the ratio of image width to image height.
- Scale the image only along X-axis or Horizontal axis. Meaning, change width, keeping height same as that of original image.
- Scale the image only along Y-axis or Vertical axis. Meaning, change height, keeping width same as that of original image.
Source Image
Consider the following image. We will use this image as input or source image in our ongoing example programs.
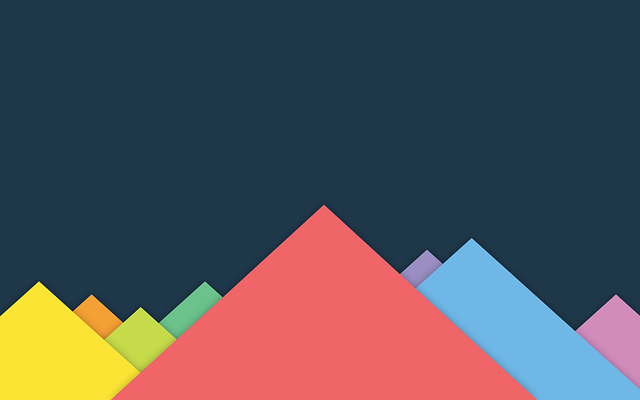
Examples
1. Resize Image using cv2.resize()
In the following example, we are going to see how we can resize the above image using cv2.resize()
while preserving the aspect ratio. We will resize the image to 50% of its actual shape, i.e., we will reduce its height to 50% of its original and width to 50% of its original.
Python Program
import cv2
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
#percent by which the image is resized
scale_percent = 50
#calculate the 50 percent of original dimensions
width = int(src.shape[1] * scale_percent / 100)
height = int(src.shape[0] * scale_percent / 100)
# dsize
dsize = (width, height)
# resize image
output = cv2.resize(src, dsize)
cv2.imwrite('D:/cv2-resize-image-50.png',output)
What have we done in the above Python program?
cv2.imread()
reads the given file incv2.IMREAD_UNCHANGED
with transparency channel (if any) and returns a numpy array with pixel values.scale_percent
is set to 50. We are going to scale the image to 50% of its original dimensions, both width and height.- src.shape[1] gives the width of the source image.
int(src.shape[1] * scale_percent / 100)
calculates 50% of the original width. Similarly height is also calculated. - Then we are setting the desired size
dsize
with the newly computedwidth
andheight
. cv2.resize
resizes the imagesrc
to the sizedsize
and returns numpy array.- Using
cv2.imwrite
, we are writing the output ofcv2.resize
to a local image file.
Output Image
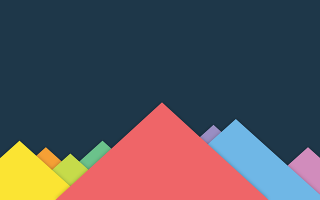
2. cv2 Resize image only horizontally
In the following example, we will scale the image only along x-axis or Horizontal axis. And we keep the height of the image unchanged.
In the dsize, we will keep the height same as that of original image but change the width.
Python Program
import cv2
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
# set a new width in pixels
new_width = 300
# dsize
dsize = (new_width, src.shape[0])
# resize image
output = cv2.resize(src, dsize, interpolation = cv2.INTER_AREA)
cv2.imwrite('D:/cv2-resize-image-width.png',output)
Explanation
- The program imports the
cv2
module from OpenCV, which is used for image processing tasks. src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
reads the input image from the specified file path. Thecv2.IMREAD_UNCHANGED
flag ensures the image is loaded with all its channels, including the alpha channel if present.- The desired width for the resized image is set to
300
pixels withnew_width = 300
. - The new image dimensions are calculated as a tuple with the new width and the original height
src.shape[0]
. This is stored indsize = (new_width, src.shape[0])
. - The function
cv2.resize(src, dsize, interpolation = cv2.INTER_AREA)
resizes the input imagesrc
to the specified dimensionsdsize
. The interpolation methodcv2.INTER_AREA
is used, which is ideal for shrinking images. - The resized image is saved to the specified file path using
cv2.imwrite('D:/cv2-resize-image-width.png', output)
.
Output Image
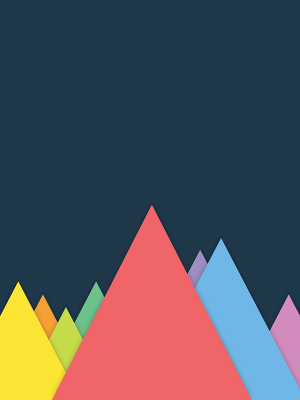
3. cv2 - Resize image only vertically
In the following example, we will scale the image only along y-axis or Vertical axis. Width of the output image remains unchanged from that of the source image.
In the dsize, we will keep the width same as that of original image but change the height.
Python Program
import cv2
src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
# set a new height in pixels
new_height = 200
# dsize
dsize = (src.shape[1], new_height)
# resize image
output = cv2.resize(src, dsize, interpolation = cv2.INTER_AREA)
cv2.imwrite('D:/cv2-resize-image-height.png',output)
Explanation
- The program imports the
cv2
module from OpenCV, which is used for image processing. src = cv2.imread('D:/cv2-resize-image-original.png', cv2.IMREAD_UNCHANGED)
reads the input image from the specified file path with thecv2.IMREAD_UNCHANGED
flag, which loads the image as is (including any alpha channel if present).- The new height for the image is set to
200
pixels withnew_height = 200
. - The program calculates the new image size using the original width
src.shape[1]
and the new height.dsize = (src.shape[1], new_height)
stores this information in a tuple representing the new dimensions. - The
output = cv2.resize(src, dsize, interpolation = cv2.INTER_AREA)
line resizes the original imagesrc
to the new dimensions stored indsize
, using thecv2.INTER_AREA
interpolation method for better quality in image reduction. - Finally, the resized image is saved to a new file path with
cv2.imwrite('D:/cv2-resize-image-height.png',output)
.
Output Image
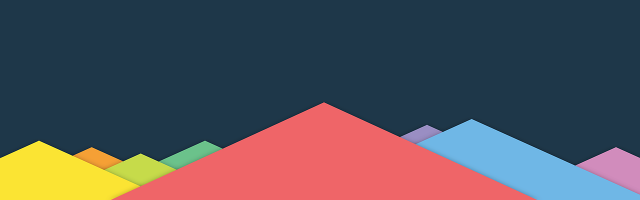
Summary
In this Python OpenCV Tutorial, we have learned how to use OpenCV cv2.resize() function, to resize an image along width, height or both by preserving the aspect ratio or not preserving the aspect ratio.