Find Contours in Image - Python OpenCV - cv2.findContours()
OpenCV - Contours of Image
Contour in image is an outline on the objects present in the image. The significance of the objects depend on the requirement and threshold you choose.
In this tutorial, we shall learn how to find contours in an image, using Python OpenCV library.
Step to find contours in an image
To find contours in an image, follow these steps:
- Read image as grey scale image.
- Use cv2.threshold() function to obtain the threshold image.
- Use cv2.findContours() and pass the threshold image and necessary parameters.
- findContours() returns contours. You can draw it on the original image or a blank image.
Examples
1. Find contours in given image
In this example, we will take the following image and apply the above said steps to find the contours.
Input Image
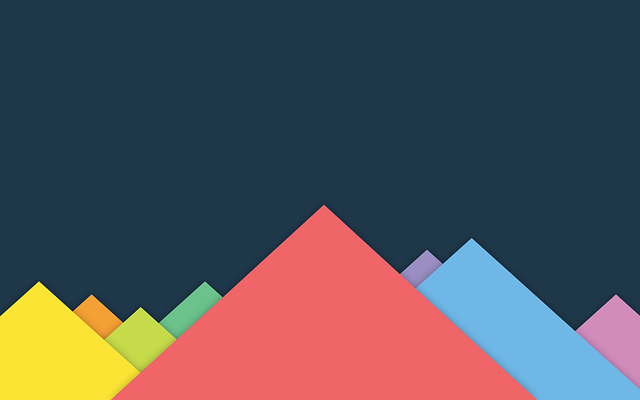
In this example, we will write the contours to a new binary image.
Python Program
import cv2
import numpy as np
img = cv2.imread('D:/original.png', cv2.IMREAD_UNCHANGED)
#convert img to grey
img_grey = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
#set a thresh
thresh = 100
#get threshold image
ret,thresh_img = cv2.threshold(img_grey, thresh, 255, cv2.THRESH_BINARY)
#find contours
contours, hierarchy = cv2.findContours(thresh_img, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
#create an empty image for contours
img_contours = np.zeros(img.shape)
# draw the contours on the empty image
cv2.drawContours(img_contours, contours, -1, (0,255,0), 3)
#save image
cv2.imwrite('D:/contours.png',img_contours)
Output Image
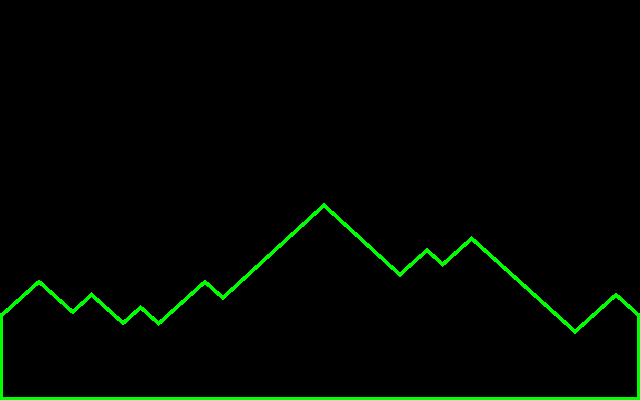
In the above python program, we have taken a threshold value of 100. If you change the threshold, the contours also change. Let us take threshold value as 128 and see the result.
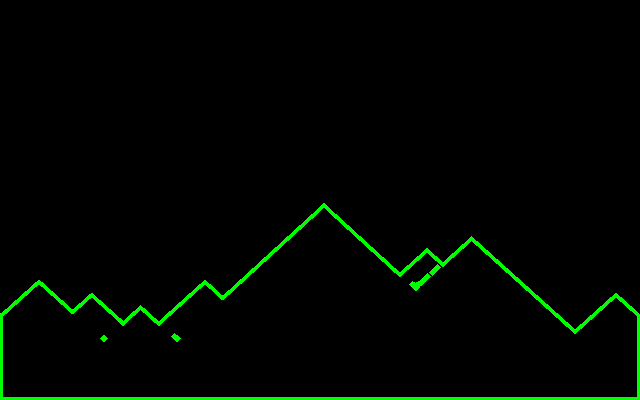
Based on the color distribution and characteristics of your source image, you have to choose a threshold value.
Summary
In this Python OpenCV Tutorial, we learned how to find contours in image using Python OpenCV library.