Matplotlib – Label Font Family
To set a specific font family for a label of the plot in Matplotlib, you can use fontfamily
parameter or, fontdict
parameter of the label functions.
In this tutorial, we shall go through both the approaches, and look into the situations when to use which.
Approach 1: Using fontfamily
parameter
This approach is used when you would like to change the font family for a specific label, when granular customization is required.
plt.xlabel('X-axis Sample Label', fontfamily='cursive')
plt.ylabel('Y-axis Sample Label', fontfamily='Futura')
X-axis label is set with a font family of ‘cursive’, and Y-axis label is set with a font family of ‘Futura’. You can also use other font family names such as ‘sans-serif’, ‘monospace’, or specific font names like ‘Times New Roman’ depending on your preference.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [10, 20, 35, 45, 30]
# Plot the data
plt.plot(x, y, marker='o')
# Set font family for the labels
plt.xlabel('X-axis Sample Label', fontfamily='cursive')
plt.ylabel('Y-axis Sample Label', fontfamily='Futura')
# Show the plot
plt.show()
Output
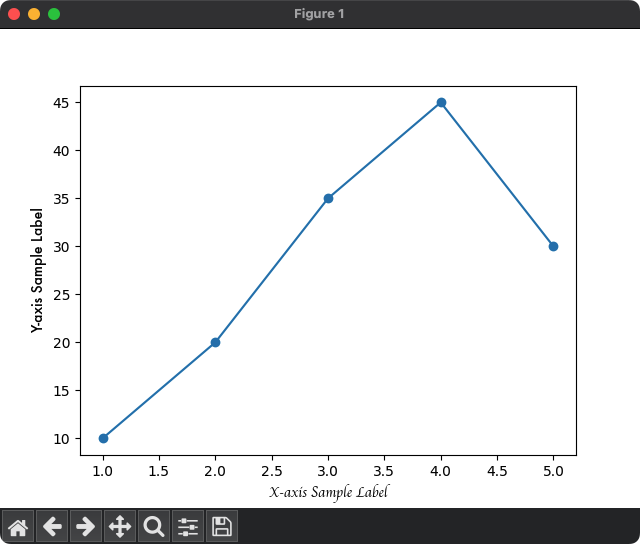
Approach 2: Using fontdict
parameter
This approach is useful when you would like to set a specific font for all the labels, like a global setting for the font, and you would like to make the code more modular. In the font dictionary object, you can also specify the color, and size in addition to the font family.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [10, 20, 35, 45, 30]
# Plot the data
plt.plot(x, y, marker='o')
# Set font family
my_font = {'family':'Futura'}
# Set fontdict for the labels
plt.xlabel('X-axis Sample Label', fontdict = my_font)
plt.ylabel('Y-axis Sample Label', fontdict = my_font)
# Show the plot
plt.show()
Output
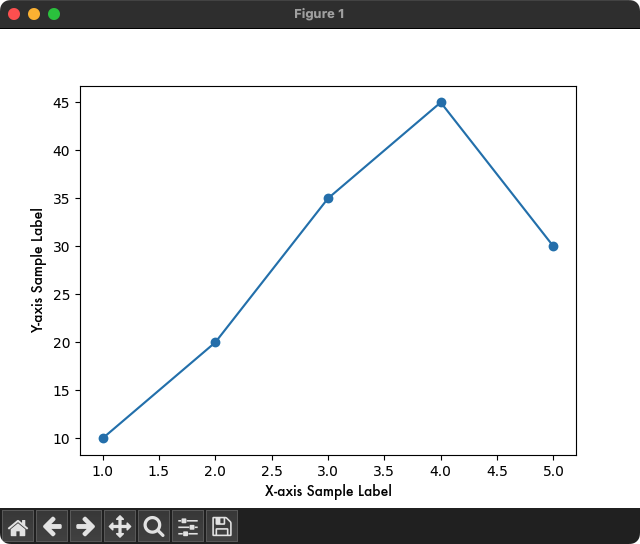