Matplotlib – Plot line
In this tutorial, we’ll create a simple line plot using Matplotlib in Python.
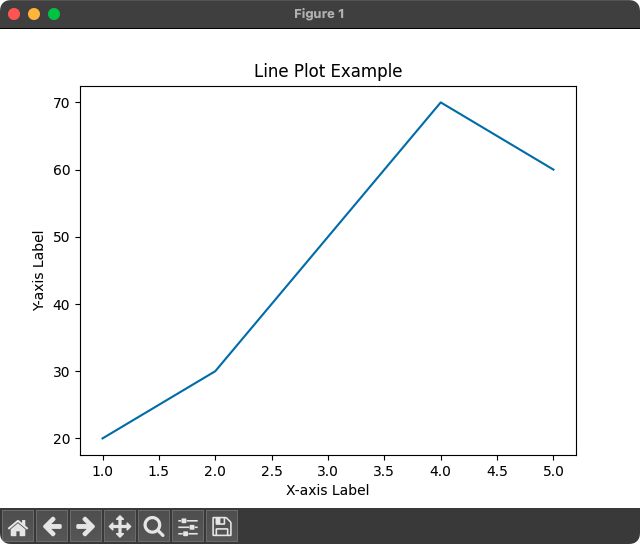
1. Import Matplotlib
Import the Matplotlib library, specifically the pyplot
module.
import matplotlib.pyplot as plt
2. Create Data
Define the data points for the X and Y axes.
x = [1, 2, 3, 4, 5]
y = [20, 30, 50, 70, 60]
In this case, x
represents the values on the X-axis, and y
represents the corresponding values on the Y-axis.
3. Plot Line
Use Matplotlib plot()
function to plot the line.
plt.plot(x, y)
4. Customize Plot
Customize the plot with labels and titles.
plt.title('Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
The title()
, xlabel()
, and ylabel()
functions set the title, X-axis label, and Y-axis label, respectively.
5. Show the Plot
Display the plot.
plt.show()
The show()
function is essential for rendering the plot and making it visible.
Complete program to plot line using Matplotlib
Using all the above steps, let us write the complete program to draw a line using Matplotlib plot() function.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 50, 70, 60]
# Plot line
plt.plot(x, y)
# Customize plot
plt.title('Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
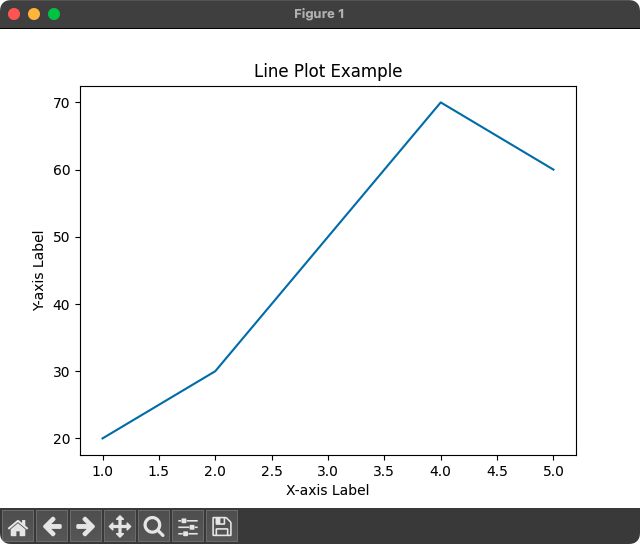
You can also specify a marker to the points using marker
parameter to the plot() function. For example, marker='o'
displays circle markers at given points.
Python Program
import matplotlib.pyplot as plt
# Example data
x = [1, 2, 3, 4, 5]
y = [20, 30, 50, 70, 60]
# Plot line
plt.plot(x, y, marker='o')
# Customize plot
plt.title('Line Plot Example')
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
# Show the plot
plt.show()
Output
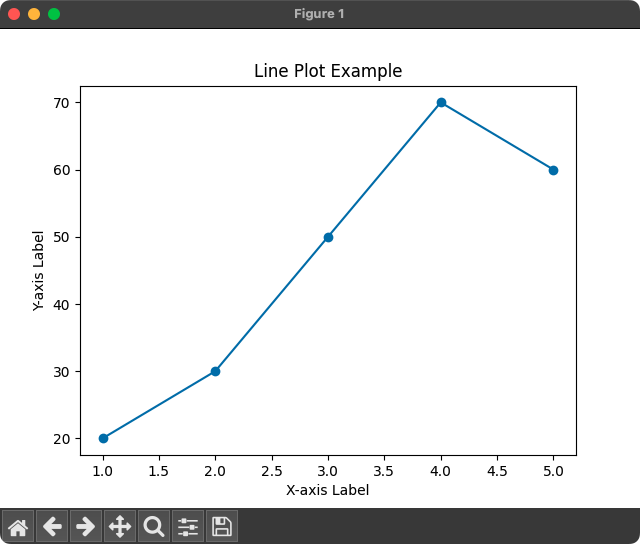
For complete list of markers, please refer Matplotlib Marker Reference.
Summary
That concludes our tutorial on creating a line plot using Matplotlib in Python!