Matplotlib – Two Scatter Plots in the Same Figure
In this tutorial, we’ll learn how to create a Matplotlib figure with two scatter plots.
The following is a step by step tutorial on how to draw two Scatter plots in the same figure using Matplotlib.
1. Import Necessary Libraries
Start by importing the required Matplotlib library. Optionally, you can also import NumPy for generating sample data.
import matplotlib.pyplot as plt
import numpy as np
2. Generate Sample Data
Create two arrays of data points for the X and Y axes for each scatter plot. For this example, we’ll use NumPy to generate random data.
# Number of data points
num_points = 50
# Generate random values for X and Y for both plots
x_values_1 = np.random.rand(num_points)
y_values_1 = np.random.rand(num_points)
x_values_2 = np.random.rand(num_points)
y_values_2 = np.random.rand(num_points)
3. Create Scatter Plots
Use Matplotlib’s scatter()
function to create scatter plots for both sets of data points.
# Create scatter plots for both datasets
plt.scatter(x_values_1, y_values_1, label='Scatter Plot 1')
plt.scatter(x_values_2, y_values_2, label='Scatter Plot 2')
4. Customize and Show the Plot
Customize the plot by adding a title, labels for the X and Y axes, and a legend. Finally, display the plot using show()
.
# Customize the plot
plt.title('Two Scatter Plots Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Complete Program for Two Scatter Plots in the same Figure
Here’s the complete program to plot two scatter plots by using all the above mentioned steps.
Python Program
import matplotlib.pyplot as plt
import numpy as np
# Number of data points
num_points = 50
# Generate random values for X and Y for both plots
x_values_1 = np.random.rand(num_points)
y_values_1 = np.random.rand(num_points)
x_values_2 = np.random.rand(num_points)
y_values_2 = np.random.rand(num_points)
# Create scatter plots for both datasets
plt.scatter(x_values_1, y_values_1, label='Scatter Plot 1')
plt.scatter(x_values_2, y_values_2, label='Scatter Plot 2')
# Customize the plot
plt.title('Two Scatter Plots Example')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Show the plot
plt.show()
Output
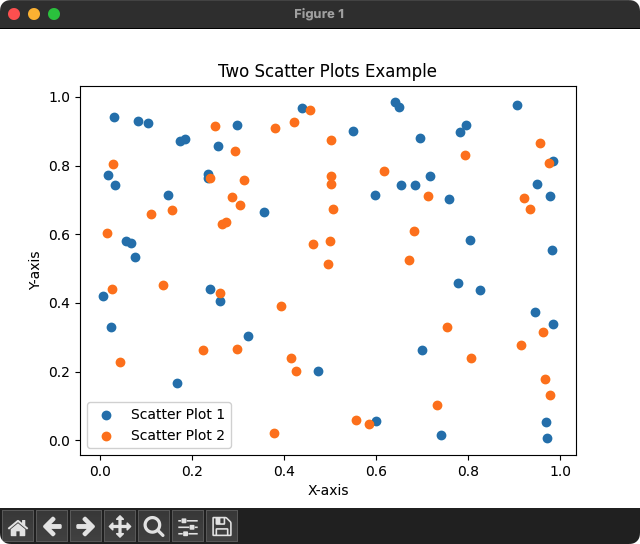
Summary
This tutorial demonstrated how to create a Matplotlib figure with two scatter plots, allowing for a visual comparison between two sets of data points.